How to Wait for Page to Load in JavaScript
- Use Event Listener to Wait for Page to Load in JavaScript
-
Use the
window.onload
Event to Wait for the Page to Load in JavaScript
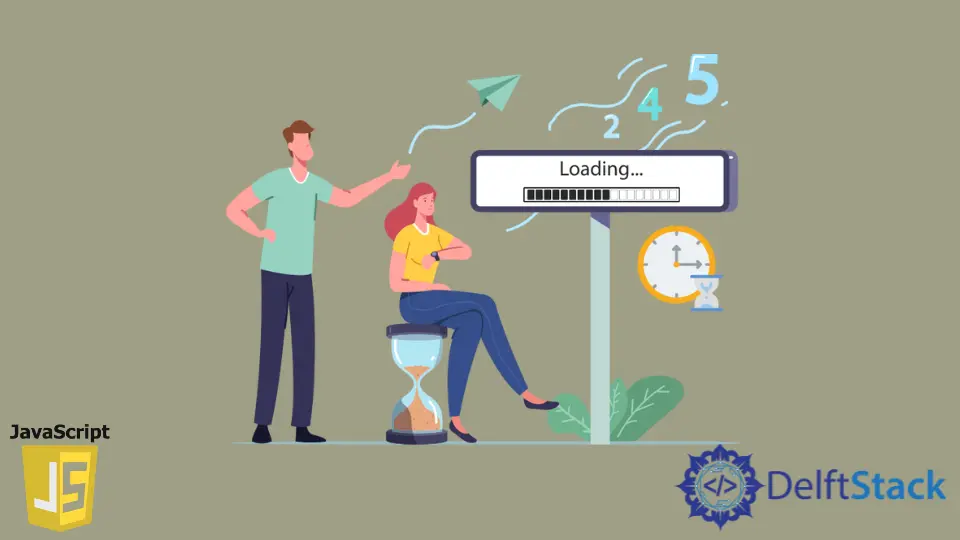
Today’s post will teach about waiting for a page to load entirely in JavaScript.
Use Event Listener to Wait for Page to Load in JavaScript
The EventTarget
interface’s addEventListener()
method configures a function to be called whenever the specified event is delivered to the target.
The addEventListener()
method works by adding a function or object that implements the EventListener
to the list of event listeners for the event type specified in the EventTarget
for which it is called.
If the function or object is already in the list of event listeners for that target, the function or object is not added a second time.
The removeEventListener()
method of the EventTarget
interface removes an event listener that was previously registered from the target using EventTarget.addEventListener()
.
Let’s take a look at the example below.
<div>
<p>
Welcome to wait for page load blog post.
</p>
</div>
window.addEventListener(
'load', () => {alert('Hello World.This page is loaded!')});
The event listener function is registered to the window in the above example. This event listener waits for the page to load completely before alerting the user that the page is loaded.
Try to run the following code in your browser; it will show the following result.
Output:
Use the window.onload
Event to Wait for the Page to Load in JavaScript
The GlobalEventHandlers
mixin’s onload
property is an event handler that handles load events in a window
, XMLHttpRequest
, img
element, etc.
The load event is triggered when a specific resource has been loaded.
The load event is triggered at the end of the document load process. All objects in the document are in the DOM, and all images, scripts, links, and subframes have fully loaded.
The sole difference between both solutions is that the window.onload
, in recent browsers, doesn’t fire window.onload
when you use the back/forward history buttons. In contrast, listeners are fired every time.
<div>
<p>
Welcome to wait for page load blog post.
</p>
</div>
window.onload = function() {
console.log('Hola!.This page is loaded!')
};
Output:
"Hola!.This page is loaded!"
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn