How to Use Variable as Key in JavaScript
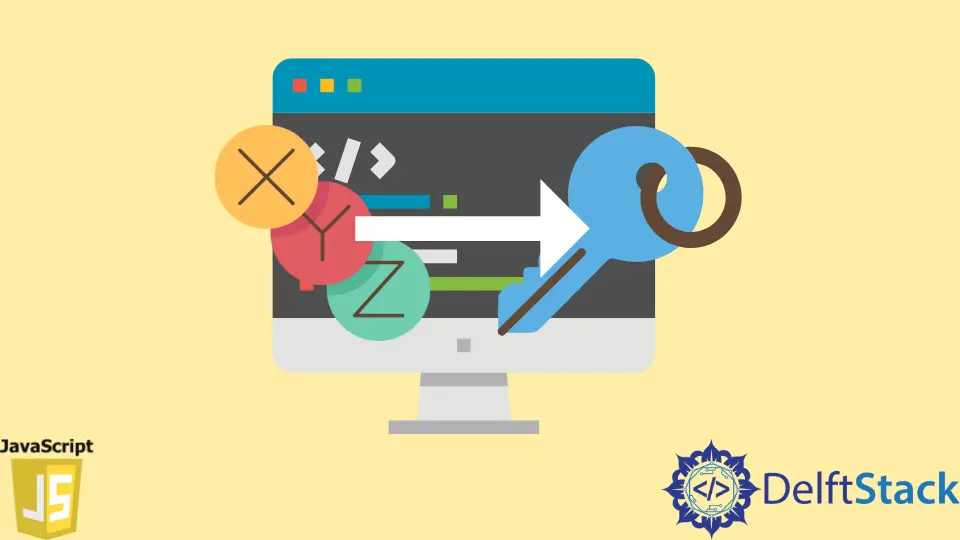
Objects follow the rule of maintaining a key-value
pair, and the key is meant to be irredundant.
To access a value of an object, we either use the dot(.)
operator, or we mention the key string within the square brackets ([])
.
But when we set a variable for the key, we can also use that according to some regulations.
Use Variable as Key for Objects in JavaScript
We will set a variable varr
and enter the key key
in this instance. Later we will define an object obj
and set the unique key in obj[varr]
.
var varr = 'key';
var obj = {};
obj[varr] = 42;
console.log(obj.key);
console.log(obj['key']);
The variable varr
was set as the key for the object obj
. The actual key is the key
stored in varr
.
But using a variable as a key means adding the variable in the square brackets along with the object.
Output:
If we wish to console out the value, you can see two ways. One is to use the dot
operator and mention the key name explicitly.
Secondly, use a square bracket
with the object but in a string form as our key
is a string type.
But you cannot access the object’s value using the variable where you stored your key
name.
Related Article - JavaScript Variable
- How to Access the Session Variable in JavaScript
- How to Check Undefined and Null Variable in JavaScript
- How to Mask Variable Value in JavaScript
- Why Global Variables Give Undefined Values in JavaScript
- How to Declare Multiple Variables in a Single Line in JavaScript
- How to Declare Multiple Variables in JavaScript