Underscore Prefix in JavaScript
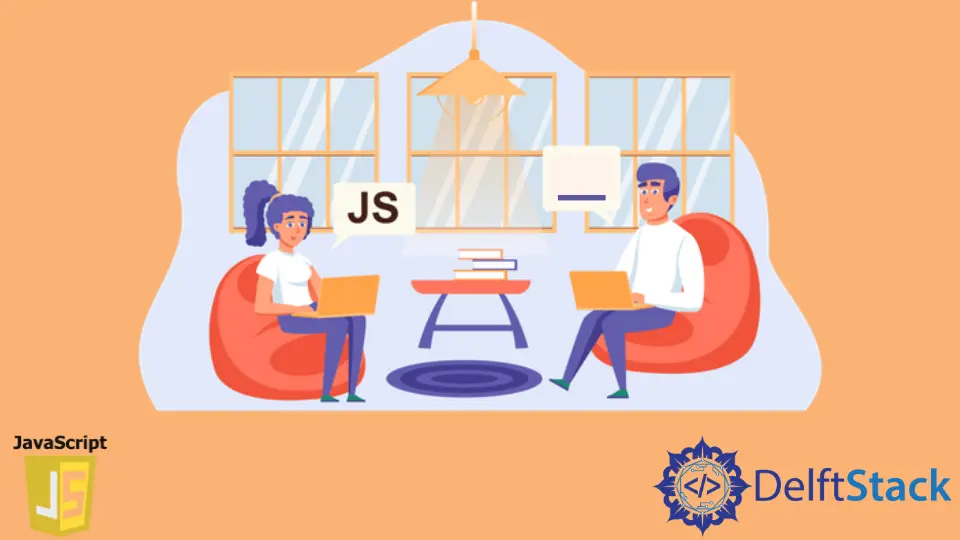
This article helps you understand the JavaScript underscore prefix in variables.
the Underscore Prefix in JavaScript Variables
The underscore _
is simply an acceptable character for variable/function names; it has no additional functionality.
Underscore variable is the standard for private variables and methods. There is no true class privacy in JavaScript.
It signifies that these methods (beginning with _
) should not be used outside your object. Technically, you can, but _
indicates that you shouldn’t.
For example, we can define a method’s name as _render
because the underscore is a permitted identifier character.
The use of underscores to designate secret methods is a typical trend in languages without access modifiers. In a language with access modifiers, such as C#, we could define a method as:
private void Soo() {}
Soo
can only be called from within the class that defined it. However, you can’t do this in JavaScript, so a typical design pattern is to prefix the method to indicate that it should be considered private.
this._Soo();
You can still call this function outside of the class declaration; however, doing so is not recommended.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn