How to Check if a Variable Is Undefined in JavaScript
- Method 1: Using the typeof Operator
- Method 2: Direct Comparison to Undefined
- Method 3: Using the void Operator
- Method 4: Using the Optional Chaining Operator
- Conclusion
- FAQ
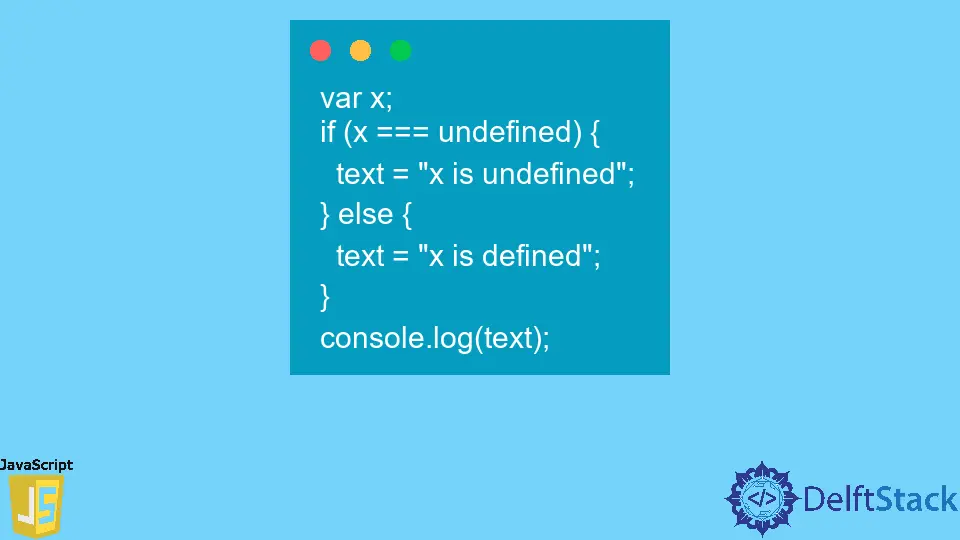
JavaScript is a versatile language, but it can sometimes lead to confusion, especially when dealing with variables. One common issue developers face is determining whether a variable is undefined. This can happen for various reasons, such as a variable not being initialized or a function not returning a value. Understanding how to check for undefined variables is crucial for writing robust and error-free code. In this tutorial, we will explore different methods to check if a variable is undefined in JavaScript, complete with code examples and detailed explanations. Whether you are a beginner or an experienced developer, this guide will help you navigate the nuances of variable checks in JavaScript.
Method 1: Using the typeof Operator
One of the simplest ways to check if a variable is undefined is by using the typeof
operator. This operator returns a string that indicates the type of the unevaluated operand. If the variable is not defined, typeof
will return the string “undefined”.
Here’s how you can use it:
let myVar;
if (typeof myVar === "undefined") {
console.log("myVar is undefined");
} else {
console.log("myVar is defined");
}
Output:
myVar is undefined
In this example, we declare a variable myVar
without assigning any value to it. When we check its type using typeof
, we find that it is indeed “undefined”. This method is particularly useful because it won’t throw an error even if the variable hasn’t been declared at all. Instead, it safely returns “undefined”, allowing you to handle such cases without causing your script to break.
Method 2: Direct Comparison to Undefined
Another straightforward method is to compare the variable directly to the undefined
keyword. This approach is simple and effective but requires that the variable has been declared. If you attempt to compare an undeclared variable directly, it will throw a ReferenceError.
Here’s how this method works:
let myVar;
if (myVar === undefined) {
console.log("myVar is undefined");
} else {
console.log("myVar is defined");
}
Output:
myVar is undefined
In this code snippet, we check if myVar
is strictly equal to undefined
. Since myVar
has been declared but not initialized, the condition evaluates to true, and the console logs that myVar is undefined
. However, be cautious when using this method; if myVar
had not been declared, the code would throw an error. Therefore, it’s typically safer to use the typeof
method in scenarios where the variable might not be declared.
Method 3: Using the void Operator
The void
operator is another interesting way to check for undefined variables. This operator evaluates an expression and then returns undefined
. You can use it to create a reliable check for whether a variable is undefined.
Here’s an example:
let myVar;
if (myVar === void 0) {
console.log("myVar is undefined");
} else {
console.log("myVar is defined");
}
Output:
myVar is undefined
In this example, we compare myVar
to void 0
. The expression void 0
always evaluates to undefined
, making it a safe way to check for undefined variables. This method is particularly useful when you want to avoid the pitfalls of directly comparing to undefined
, especially in environments where the global undefined
variable might be accidentally modified. The void
operator provides a level of safety that can be beneficial in certain coding circumstances.
Method 4: Using the Optional Chaining Operator
With the introduction of ES2020, the optional chaining operator (?.
) allows you to safely access deeply nested properties of an object without having to check for the existence of each property in the chain. If any part of the chain is undefined
, the expression will short-circuit and return undefined
, rather than throwing an error.
Here’s how to use it:
let obj = {
prop: {
nested: undefined
}
};
if (obj.prop?.nested === undefined) {
console.log("nested is undefined");
} else {
console.log("nested is defined");
}
Output:
nested is undefined
In this example, we use optional chaining to check if nested
is undefined. If obj.prop
is not defined, the expression will return undefined
without throwing an error. This method is incredibly useful when working with complex objects where properties may or may not exist, making your code cleaner and more maintainable.
Conclusion
Understanding how to check if a variable is undefined in JavaScript is essential for writing efficient and error-free code. We explored several methods, including using the typeof
operator, direct comparison, the void
operator, and the optional chaining operator. Each method has its advantages and can be used in different scenarios based on your specific needs. By mastering these techniques, you can enhance your coding skills and create more robust applications.
FAQ
-
What does it mean when a variable is undefined?
A variable is considered undefined when it has been declared but has not been assigned a value. -
Can I check for undefined variables without declaring them?
Yes, using thetypeof
operator allows you to check for undefined variables without throwing an error. -
Is it safe to compare a variable directly to undefined?
It is safe only if the variable has been declared; otherwise, it will throw a ReferenceError. -
What is the optional chaining operator?
The optional chaining operator allows you to safely access deeply nested properties of an object without causing an error if a property is undefined. -
Why use the void operator?
The void operator can be used to create a reliable check for undefined variables, providing a safeguard against accidental modifications to the global undefined variable.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedInRelated Article - JavaScript Variable
- How to Access the Session Variable in JavaScript
- How to Check Undefined and Null Variable in JavaScript
- How to Mask Variable Value in JavaScript
- Why Global Variables Give Undefined Values in JavaScript
- How to Declare Multiple Variables in a Single Line in JavaScript
- How to Declare Multiple Variables in JavaScript