JavaScript Uncaught TypeError
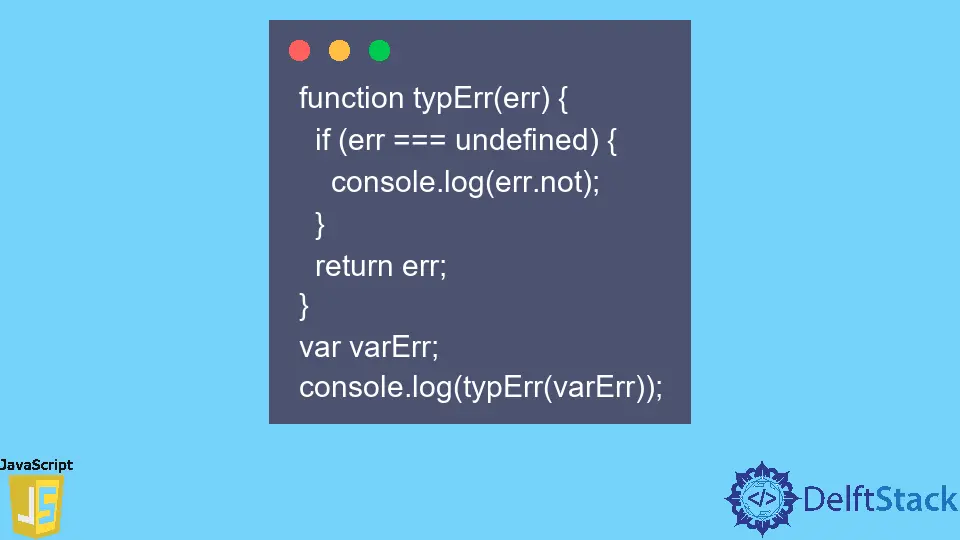
This article will help you understand the Uncaught TypeError
and how to solve it in JavaScript.
Fix the Uncaught TypeError
in JavaScript
When an expression or statement is evaluated, and the type of the expression or statement is not consistent with the anticipated type, a TypeError
is raised. JavaScript can produce this error when attempting to use a value when a different type is needed, such as using a number instead of a string.
When an operand or argument provided to a function is not of the type anticipated by that operator or function, JavaScript throws a TypeError
. This error will appear when you read a property or invoke a method on an undefined object in the Chrome browser.
Depending on the type of property you are trying to access, there are a few different variants of this issue. Sometimes, it will say null
rather than undefined
.
function typErr(err) {
if (err === undefined) {
console.log(err.not);
}
return err;
}
var varErr;
console.log(typErr(varErr));
Output:
When you execute this code, your browser will display the following message:
"Uncaught TypeError: Cannot read properties of undefined (reading 'not')"
Undefined, in its simplest form, denotes a variable that has been declared but has not been given a value. Since undefined is not an object type (but rather its undefined type), since properties may only belong to objects in JavaScript, undefined is perhaps the best perspective from which to analyze this specific problem.
If you see an undefined error in the code above, make sure that the variable that is to blame has a value given to it.
function typErr(err) {
if (err === undefined) {
console.log(err.not);
}
return err;
}
var varErr = 100;
console.log(typErr(varErr));
The variable varErr
is initialized at 100 in the JavaScript shown above. The script so executed correctly.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn