JavaScript Tuple Example
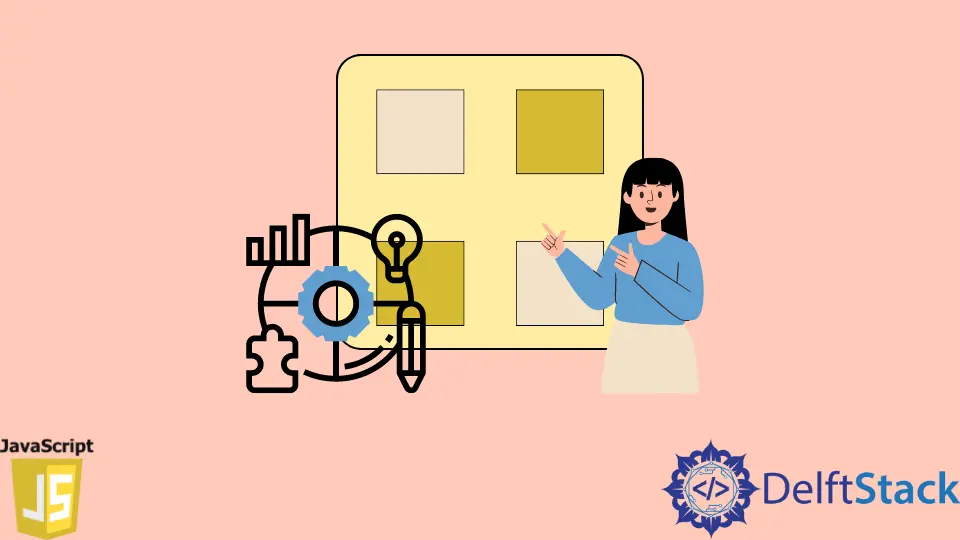
In JavaScript language, tuples are types of array with immutable features. We can access tuple with a single variable which is a type of an array
.
Tuple in JavaScript
class MyBestTupleExplanation extends Array {
constructor(...items) {
super(...items);
Object.freeze(this);
}
}
let tuple = new MyBestTupleExplanation('Afridi', 35, 'White');
let [myName, myAge, skinColor] = tuple;
console.debug(myName); // Afridi
console.debug(myAge); // 35
console.debug(skinColor); // White
console.debug('******************************************');
console.debug('Now we are re-assigning the values to tuple');
console.debug('******************************************');
tuple = ['Bob', 24]; // no effect
console.debug(myName); // Afridi
console.debug(myAge); // 35
console.debug(skinColor); // White
Output:
Afridi
35
White
******************************************
Now we are re-assigning the values to tuple
******************************************
Afridi
35
White
In the above example, we created a class named MyBestTupleExplanation
, inherited from the Array
class. It will behave like an array but with immutability features. In this class, we call the constructor of the Array
class with the super
method.
In the above code, we used the MyBestTupleExplanation
class, and in its constructor, we called the method freeze
from parent class Object
and passed an array to it.
After creating this class, we have created the object of that class named MyBestTupleExplanation
and passed the values through the constructor.
We have created an anonymous array of variables and assigned our tuple to that array. The elements in the tuple will be assigned index-wise to the array
. It will not work if we reassign the values to that tuple later. As shown in the example, we can not reassign the values to the tuple.
JavaScript Tuple Example 2
class MyBestTupleExplanation extends Array {
constructor(...items) {
super(...items);
Object.freeze(this);
}
}
let tuple = new MyBestTupleExplanation('Afridi', 35);
let [myName, myAge, skinColor] = tuple;
console.debug(myName); // Afridi
console.debug(myAge); // 35
console.debug(skinColor); // undefined
Output:
Afridi
35
undefined
In the above example, we have created a variable skinColor
but not initializing its value; that’s why we are getting an undefined error.