How to Scroll A Table in JavaScript
-
Use the
overflow
Property in JavaScript to Scroll Table -
Use
overflowX
andoverflowY
in JavaScript to Scroll Through Table
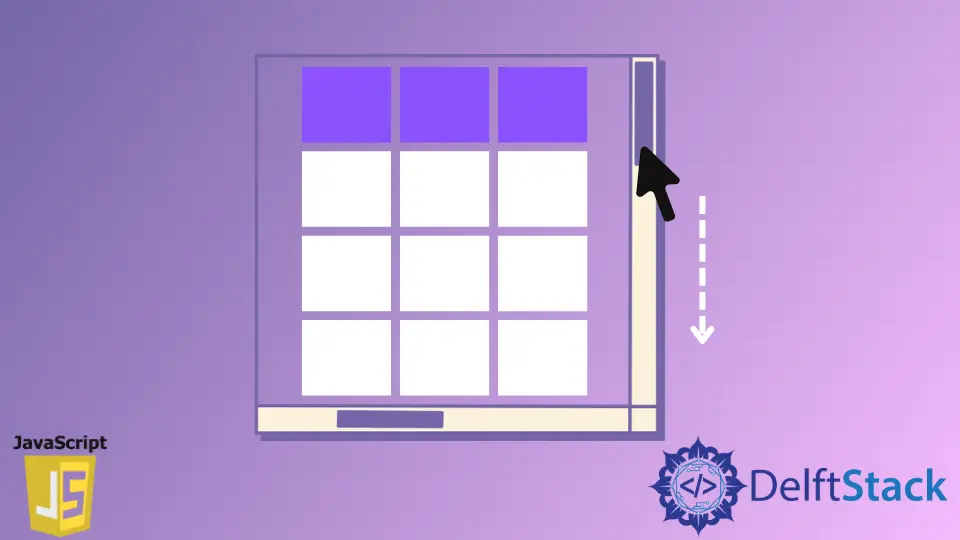
For web development and utilizing the spaces of a webpage, scrollable content or div or table can actively contribute. A scrollable section can easily save a large pile of lines and can redesign the webpage.
Our example sets will demonstrate the use of the overflow: "auto"
and the overflowX, overflowY
properties. These properties require fewer lines of code to implement scrollable table content.
Use the overflow
Property in JavaScript to Scroll Table
The basic structure for the table
gives a large list of data. We will initiate a scrollable table to minimize the space and utilize page areas.
We will set the tbody
instance property overflow
to auto
. It will automatically pop up a navigator that moves vertically and horizontally.
Code - HTML File:
<table id="myTable">
<thead>
<tr>
<th>Month</th>
<th>Savings</th>
</tr>
</thead>
<tbody id="bd">
<tr>
<td>January</td>
<td>$100</td>
</tr>
<tr>
<td>February</td>
<td>$80</td>
</tr>
<tr>
<td>January</td>
<td>$100</td>
</tr>
<tr>
<td>February</td>
<td>$80</td>
</tr>
<tr>
<td>January</td>
<td>$100</td>
</tr>
<tr>
<td>February</td>
<td>$80</td>
</tr>
</tbody>
</table>
Code - CSS File:
thead,
tbody {
display: block;
}
tbody {
max-height: 100px;
width: 200px;
}
Code - JavaScript File:
document.getElementById('bd').style.overflow = 'auto';
Output:
Use overflowX
and overflowY
in JavaScript to Scroll Through Table
This example implies an almost similar way of performing the task of making a scrollable table. The difference is that we define the axis navigation explicitly.
We will set the overflowX
to none
and overflowY
to auto
.
Code - HTML File:
<table id="myTable">
<thead>
<tr>
<th>Month</th>
<th>Savings</th>
</tr>
</thead>
<tbody id="bd">
<tr>
<td>January</td>
<td>$100</td>
</tr>
<tr>
<td>February</td>
<td>$80</td>
</tr>
<tr>
<td>January</td>
<td>$100</td>
</tr>
<tr>
<td>February</td>
<td>$80</td>
</tr>
<tr>
<td>January</td>
<td>$100</td>
</tr>
<tr>
<td>February</td>
<td>$80</td>
</tr>
</tbody>
</table>
Code - CSS File:
thead,
tbody {
display: block;
}
tbody {
max-height: 100px;
width: 200px;
}
Code - JavaScript File:
document.getElementById('bd').style.overflowX = 'none';
document.getElementById('bd').style.overflowX = 'auto';
Output: