How to Switch String in JavaScript
- Understanding the Switch-Case Statement
- Example of Switch-Case with Strings
- Using Multiple Cases in a Switch Statement
- Best Practices for Using Switch Statements
- Conclusion
- FAQ
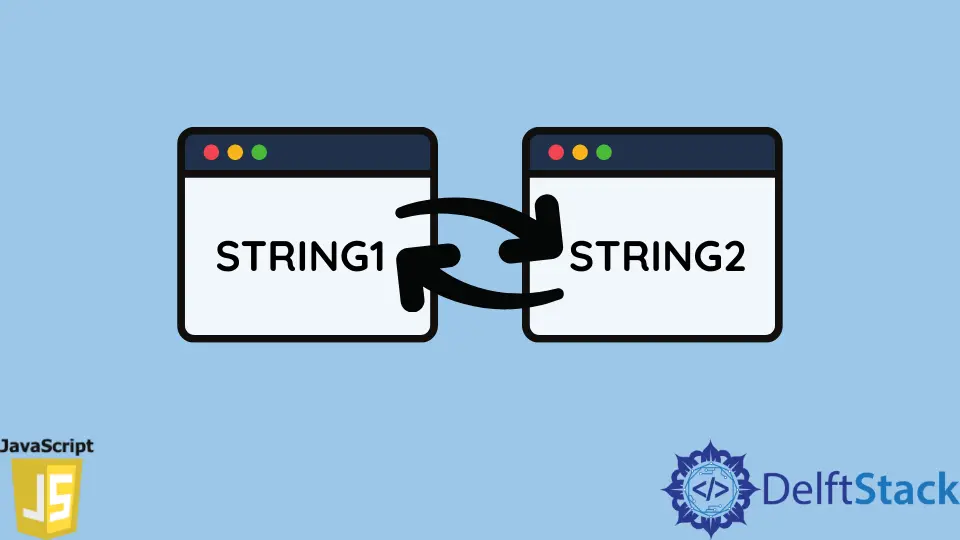
Switch-case statements in JavaScript are powerful tools that allow developers to evaluate an expression and execute different blocks of code based on the result. This structured approach is particularly useful when you have multiple conditions to check against a single variable. Instead of using several if-else statements, a switch-case can make your code cleaner and easier to read.
In this article, we will explore how to implement the switch-case statement in JavaScript, including examples and detailed explanations. Whether you are new to programming or looking to refine your skills, understanding how to switch strings in JavaScript is essential for writing efficient code.
Understanding the Switch-Case Statement
The switch-case statement starts with the keyword switch
, followed by an expression in parentheses. This expression is evaluated, and the result is compared against the values specified in the case clauses. If a match is found, the corresponding block of code executes. If no match is found, the default
case, if provided, will execute.
Here’s a simple structure of a switch-case statement:
switch (expression) {
case value1:
// code block
break;
case value2:
// code block
break;
default:
// default code block
}
In this structure, each case
represents a potential match for the expression. The break
statement is crucial as it prevents the execution from falling through to subsequent cases. If no cases match, the code within the default
block will execute.
Example of Switch-Case with Strings
Let’s take a look at a practical example of how to use a switch-case statement with strings in JavaScript. In this example, we will evaluate a variable representing the day of the week and print out a corresponding message.
let day = "Monday";
switch (day) {
case "Monday":
console.log("Start of the work week!");
break;
case "Wednesday":
console.log("Midweek day!");
break;
case "Friday":
console.log("Almost the weekend!");
break;
default:
console.log("Just another day!");
}
Output:
Start of the work week!
In this code snippet, we define a variable day
with the value “Monday”. The switch-case statement evaluates this variable, and since it matches the first case, it executes the corresponding code block, printing “Start of the work week!” to the console. If day
had a different value, the switch-case would check the subsequent cases until it finds a match or defaults to the last case.
Using Multiple Cases in a Switch Statement
You can also group multiple cases together in a switch statement. This is particularly useful when you want to execute the same block of code for different values. Let’s see how that works:
let fruit = "Apple";
switch (fruit) {
case "Apple":
case "Banana":
console.log("This is a fruit.");
break;
case "Carrot":
console.log("This is a vegetable.");
break;
default:
console.log("Unknown food item.");
}
Output:
This is a fruit.
In this example, both “Apple” and “Banana” lead to the same output. When fruit
is evaluated, it matches the first case, and the console logs “This is a fruit.” This feature allows for cleaner code when multiple values share the same outcome.
Best Practices for Using Switch Statements
While switch-case statements can simplify your code, there are best practices to keep in mind to enhance readability and maintainability. Here are a few tips:
- Use Break Statements: Always include break statements to prevent unintended fall-through behavior.
- Default Case: Always consider including a default case to handle unexpected values.
- Consistent Data Types: Ensure that the expression and case values are of the same data type to avoid unexpected results.
- Limit Cases: If you find yourself with too many cases, consider whether a different structure, like an object or a map, might be more appropriate.
By following these best practices, you can effectively leverage switch-case statements in your JavaScript applications.
Conclusion
Switch-case statements in JavaScript are a powerful feature that allows for cleaner and more organized code when dealing with multiple conditional checks. By understanding how to implement and utilize this structure effectively, you can enhance your programming skills and write more efficient JavaScript code. Whether you’re building simple applications or complex systems, mastering the switch-case statement is essential for any developer.
FAQ
-
What is a switch-case statement in JavaScript?
A switch-case statement is a control structure that allows you to evaluate an expression and execute different code blocks based on its value. -
How does the switch-case statement differ from if-else statements?
The switch-case statement is often cleaner and more readable when checking a single variable against multiple potential values, whereas if-else statements can be more flexible for complex conditions. -
Do I need to include a break statement in a switch-case?
Yes, including a break statement is important to prevent fall-through behavior, where subsequent cases execute unintentionally. -
Can I use non-string values in a switch-case statement?
Yes, switch-case statements can evaluate strings, numbers, and even expressions, as long as the case values match the type of the evaluated expression. -
When should I use a switch-case statement instead of if-else?
Use a switch-case statement when you have multiple discrete values to check against a single variable, as it can improve clarity and maintainability of your code.