How to Subtract Dates in JavaScript
- Understanding JavaScript Date Objects
-
Subtracting Dates Using the
getTime()
Method - Calculating the Difference in Hours, Minutes, and Seconds
- Using Date Libraries for Enhanced Functionality
- Conclusion
- FAQ
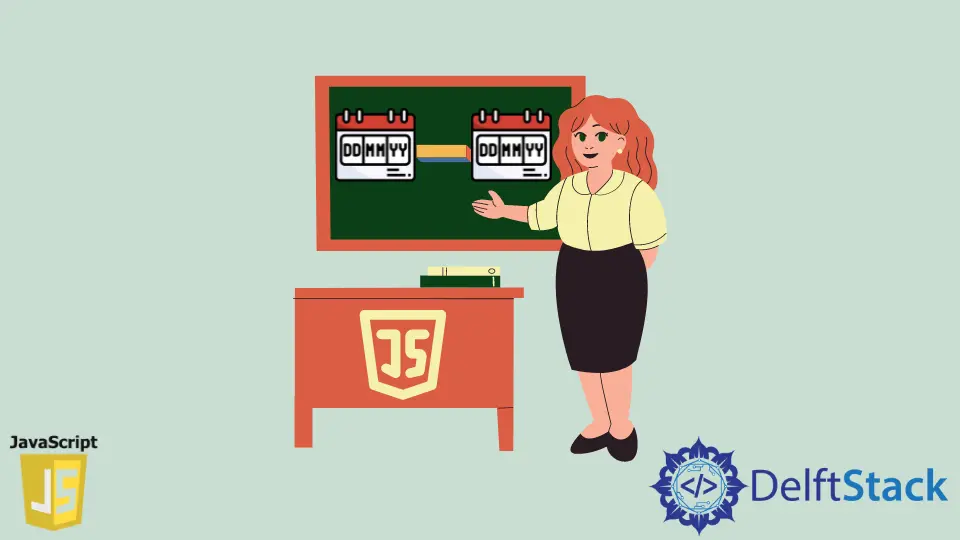
Calculating the difference between two dates is a common task in programming, especially in JavaScript. Whether you’re building a countdown timer, scheduling events, or simply tracking time, understanding how to manipulate dates can significantly enhance your projects.
In this article, we’ll explore various methods to subtract dates in JavaScript using simple functions and variables. You’ll learn how to get the difference in days, hours, minutes, and seconds, making your code more efficient and your applications more robust. So, let’s dive into the world of date manipulation in JavaScript!
Understanding JavaScript Date Objects
Before we jump into subtracting dates, it’s essential to understand how JavaScript handles dates. The JavaScript Date object is a built-in object that allows you to work with dates and times. It provides methods to create, manipulate, and format dates.
To create a new date, you can use the following syntax:
const date1 = new Date('2023-10-01');
const date2 = new Date('2023-10-15');
In this example, date1
is set to October 1, 2023, and date2
is set to October 15, 2023. With these date objects, we can perform various calculations to find the difference between them.
Subtracting Dates Using the getTime()
Method
One of the simplest ways to find the difference between two dates in JavaScript is by using the getTime()
method. This method returns the number of milliseconds since January 1, 1970, for a specified date. By subtracting the two date objects, we can easily compute the difference.
Here’s how you can do it:
const date1 = new Date('2023-10-01');
const date2 = new Date('2023-10-15');
const differenceInMilliseconds = date2.getTime() - date1.getTime();
const differenceInDays = differenceInMilliseconds / (1000 * 3600 * 24);
console.log(differenceInDays);
Output:
14
In this example, we first create two date objects. We then use the getTime()
method to retrieve the time in milliseconds for both dates. By subtracting date1
from date2
, we get the difference in milliseconds. Finally, we convert this difference into days by dividing by the number of milliseconds in a day (1000 milliseconds * 3600 seconds * 24 hours). The output shows that there are 14 days between October 1 and October 15, 2023.
Calculating the Difference in Hours, Minutes, and Seconds
If you need more detailed information about the difference between two dates, you can extend the previous method to calculate the difference in hours, minutes, and seconds. Here’s how you can do it:
const date1 = new Date('2023-10-01T12:00:00');
const date2 = new Date('2023-10-15T15:30:00');
const differenceInMilliseconds = date2.getTime() - date1.getTime();
const differenceInSeconds = Math.floor(differenceInMilliseconds / 1000);
const differenceInMinutes = Math.floor(differenceInSeconds / 60);
const differenceInHours = Math.floor(differenceInMinutes / 60);
const differenceInDays = Math.floor(differenceInHours / 24);
console.log(`Difference in Days: ${differenceInDays}`);
console.log(`Difference in Hours: ${differenceInHours}`);
console.log(`Difference in Minutes: ${differenceInMinutes}`);
console.log(`Difference in Seconds: ${differenceInSeconds}`);
Output:
Difference in Days: 14
Difference in Hours: 79
Difference in Minutes: 4770
Difference in Seconds: 286200
In this code, we create two date objects with specific times. After calculating the difference in milliseconds, we convert it to seconds, minutes, and hours. This method gives you a comprehensive view of the time difference, allowing you to present the data in a user-friendly format. The output indicates that there are 14 days, 79 hours, 4770 minutes, and 286200 seconds between the two dates.
Using Date Libraries for Enhanced Functionality
While JavaScript’s built-in Date object is powerful, you might find it beneficial to use libraries like Moment.js or date-fns for more complex date manipulations. These libraries simplify date calculations and formatting, making your code cleaner and easier to manage. Here’s a quick example using Moment.js:
const moment = require('moment');
const date1 = moment('2023-10-01');
const date2 = moment('2023-10-15');
const differenceInDays = date2.diff(date1, 'days');
const differenceInHours = date2.diff(date1, 'hours');
const differenceInMinutes = date2.diff(date1, 'minutes');
console.log(`Difference in Days: ${differenceInDays}`);
console.log(`Difference in Hours: ${differenceInHours}`);
console.log(`Difference in Minutes: ${differenceInMinutes}`);
Output:
Difference in Days: 14
Difference in Hours: 336
Difference in Minutes: 20160
In this example, we use Moment.js to create date objects and calculate the differences. The diff()
method allows you to specify the unit of time you want to measure, making it incredibly versatile. The output shows the same differences as before but with less code, enhancing readability and maintainability.
Conclusion
Subtracting dates in JavaScript is a straightforward task that can be accomplished using various methods. Whether you choose to use the built-in Date object, or enhance your capabilities with libraries like Moment.js, understanding how to manipulate dates is crucial for any developer. By applying the techniques discussed in this article, you can confidently handle date calculations in your projects, ensuring they are both efficient and user-friendly.
FAQ
-
How do I subtract two dates in JavaScript?
You can subtract two dates in JavaScript by using thegetTime()
method to get the difference in milliseconds, then converting it to days, hours, or minutes as needed. -
Can I use libraries for date manipulation in JavaScript?
Yes, libraries like Moment.js and date-fns simplify date manipulations and provide additional functionality for formatting and calculating differences. -
What is the output format when subtracting dates?
The output format can vary depending on how you choose to present the data, but common formats include days, hours, minutes, and seconds. -
Are there any performance considerations when using date libraries?
While libraries provide convenience, they may introduce additional overhead. For simple date calculations, the built-in Date object is often sufficient. -
How do I handle time zones when subtracting dates?
When working with different time zones, it’s crucial to ensure that both dates are in the same timezone or use libraries that handle time zones effectively.