How to Simulate a Struct in JavaScript
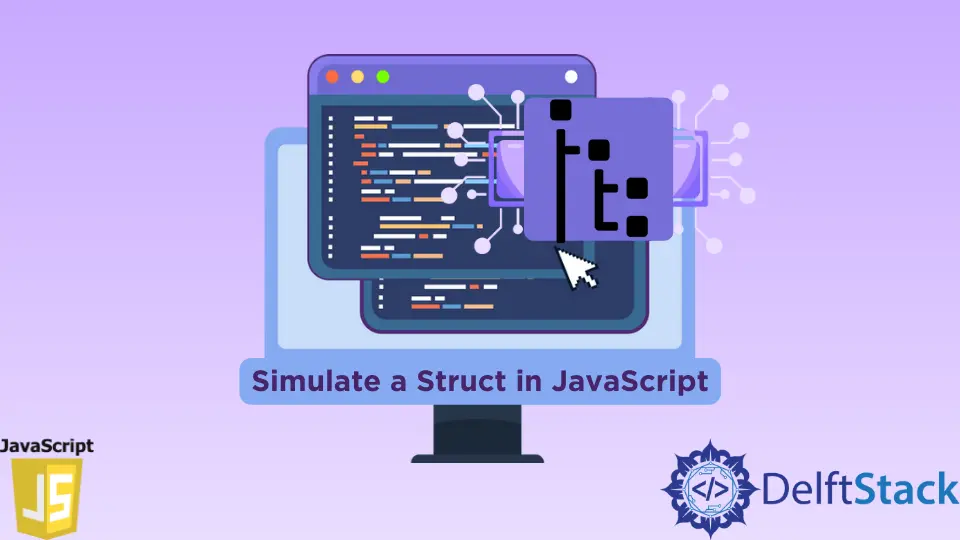
This tutorial introduces how to simulate a struct in JavaScript. In JavaScript, all the functions are objects and can have properties or variables attached to them. So, we can simulate a struct by declaring a function and attaching various properties to it.
function Movie(title, href, rating, description) {
this.title = title;
this.url = url;
this.rating = rating;
this.description = description;
}
var movies = [
new Movie('Stuck in Love', 'stuck.in.love', '4.5', ' wefwg wr w g'),
new Movie('Emily in Paris', 'emily.in.paris', '7.1', 'fgfdsadf ef wgf ')
];
Alternatively, we can also use object literals, but then we won’t be able to see all the properties attached to an object or have a common structure for all the elements by default, like a struct in C.
{ id: 1, speaker: 'john', country: 'au' } // Object Literal
The first approach is more like a struct
factory, we can create as many copies as we want, but in the case of object literals, we have to write a complete declaration again.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn