How to Strip the HTML Content Using JavaScript
- Method 1: Using the innerHTML Property
- Method 2: Using Regular Expressions
- Method 3: Using the DOMParser API
- Conclusion
- FAQ
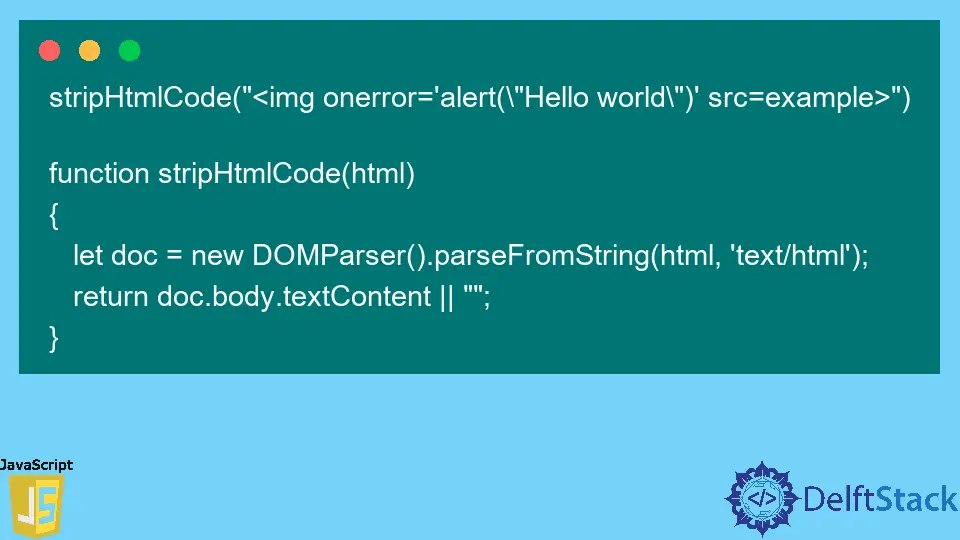
In today’s post, we’ll learn how to strip HTML content using JavaScript. Whether you’re a web developer or a beginner looking to enhance your skills, understanding how to manipulate HTML elements is crucial. Stripping HTML can be useful for various reasons, such as cleaning up user input, preparing data for storage, or simply extracting text from a webpage. JavaScript provides powerful methods that allow you to easily remove HTML tags and retrieve plain text.
In this article, we’ll explore several techniques to achieve this, ensuring you have a solid foundation to work from. Let’s dive in!
Method 1: Using the innerHTML Property
One of the simplest ways to strip HTML content is by using the innerHTML
property. This method involves creating a temporary DOM element, setting its innerHTML
to the HTML string you want to clean, and then retrieving the text content using the textContent
property.
Here’s how it works:
function stripHTML(htmlString) {
const tempDiv = document.createElement('div');
tempDiv.innerHTML = htmlString;
return tempDiv.textContent || tempDiv.innerText || "";
}
const htmlContent = "<p>Hello, <strong>world!</strong> This is a <a href='#'>link</a>.</p>";
const strippedContent = stripHTML(htmlContent);
console.log(strippedContent);
Output:
Hello, world! This is a link.
This method is effective because it leverages the browser’s ability to parse HTML. By creating a temporary div
element, we can safely manipulate the HTML without affecting the actual document. The textContent
property retrieves all the text contained within the div, effectively stripping away any HTML tags. This technique is straightforward and works well for most HTML content.
Method 2: Using Regular Expressions
Another method to strip HTML tags is by using regular expressions. This approach is less reliable than the previous one, as it can fail with complex HTML structures. However, it can be useful for quick tasks or when working with simple HTML strings.
Here’s an example of how to do it:
function stripHTMLUsingRegex(htmlString) {
return htmlString.replace(/<[^>]*>/g, '');
}
const htmlContent = "<div>Welcome to <em>JavaScript</em> programming!</div>";
const strippedContent = stripHTMLUsingRegex(htmlContent);
console.log(strippedContent);
Output:
Welcome to JavaScript programming!
In this example, the stripHTMLUsingRegex
function uses a regular expression to match and remove any HTML tags. The pattern <[^>]*>
matches anything that starts with <
, ends with >
, and contains any characters in between. While this method is quick, be cautious when using it on complex HTML, as it may lead to unexpected results.
Method 3: Using the DOMParser API
The DOMParser API is another powerful way to strip HTML content. This method allows you to parse a string of HTML into a DOM tree, from which you can easily extract text content.
Here’s how you can implement this:
function stripHTMLWithDOMParser(htmlString) {
const parser = new DOMParser();
const doc = parser.parseFromString(htmlString, 'text/html');
return doc.body.textContent || "";
}
const htmlContent = "<h1>Welcome</h1><p>This is a paragraph with <a href='#'>links</a>.</p>";
const strippedContent = stripHTMLWithDOMParser(htmlContent);
console.log(strippedContent);
Output:
Welcome
This is a paragraph with links.
Using the DOMParser API is a robust solution for stripping HTML. It allows you to handle complex HTML structures without the risk of missing nested tags or improperly formatted content. The parseFromString
method creates a new document from the HTML string, and you can easily access the text content through doc.body.textContent
. This method is highly recommended for more complex scenarios.
Conclusion
Stripping HTML content using JavaScript is an essential skill for any web developer. Whether you choose to use the innerHTML
property, regular expressions, or the DOMParser API, each method has its advantages and specific use cases. Understanding these techniques will empower you to handle HTML content more effectively, making your web applications cleaner and more efficient. So go ahead, experiment with these methods, and enhance your JavaScript skills!
FAQ
-
What is the best method to strip HTML content?
The best method depends on your specific needs. TheinnerHTML
property and DOMParser API are generally more reliable than using regular expressions. -
Can I use these methods on server-side JavaScript?
These methods are primarily designed for client-side JavaScript. For server-side, consider using libraries like Cheerio for Node.js. -
Are there any performance concerns with these methods?
For small HTML strings, performance is generally not an issue. However, for large documents, using the DOMParser API may be more efficient. -
Can I strip HTML from user input?
Yes, you can use these methods to sanitize user input before processing or storing it. -
Is it safe to use regular expressions for stripping HTML?
While regular expressions can work for simple cases, they may fail with complex or malformed HTML. It’s best to use DOM manipulation methods for reliability.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn