How to Do String Interpolation in JavaScript
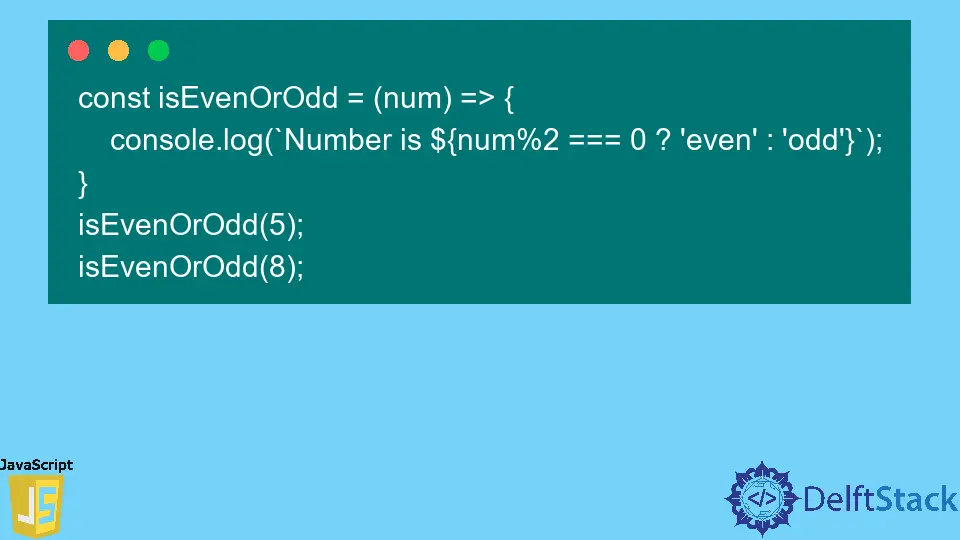
JavaScript has a great feature called string interpolation that allows you to inject a variable, a function call, and an arithmetic expression directly into a string.
In this article, we will introduce how to do string interpolation. We will have code examples below, which you can run on your machine.
Use a New Feature of ES6 to Do String Interpolation in JavaScript
Before the release of ES6, string interpolation was not available in JavaScript. The lack of this feature led to string concatenation code, as shown below.
Example:
const generalInformation = (firstName, lastName, Country) => {
return 'First Name ' + firstName + ' Last Name: ' + lastName + '. ' +
Country + ' is my country.';
} console.log(generalInformation('Mark', 'John', 'US'));
Output:
First Name Mark Last Name: John. US is my country.
String interpolation is a feature that allows you to inject variables, function calls, and arithmetic expressions directly into a string without using concatenation or escape character for multi-line strings.
We use backticks for template literals in string interpolation and use the format - ${ourValue}
to insert dynamic values like variables, function calls, and arithmetic expressions.
Example:
const generalInformation = (firstName, lastName, Country) => {
return `First Name: ${firstName} Last Name: ${lastName}. Country: ${Country}`;
} console.log(generalInformation('Mark', 'John', 'US'));
Output:
First Name: Mark Last Name: John. Country: US
We know now how a fantastic feature the string interpolation is
Example:
Let’s inject a function call and an arithmetic expression.
const generalInformation = (firstName, lastName, Country) => {
return `First Name: ${firstName} Last Name: ${lastName}. Country: ${Country}`;
} console.log(generalInformation('Mark', 'John', 'US'));
console.log(`${generalInformation("Mark", "John", "US")} He is a Worker in our company.`);
console.log(`sum of 10 and 6 is ${10+6}.`);
Output:
First Name: Mark Last Name: John. Country: US
First Name: Mark Last Name: John. Country: US He is a Worker in our company.
sum of 10 and 6 is 16.
Example:
We can use conditional statements in string interpolation.
const isEvenOrOdd = (num) => {
console.log(`Number is ${num % 2 === 0 ? 'even' : 'odd'}`);
} isEvenOrOdd(5);
isEvenOrOdd(8);
Output:
Number is odd
Number is even