How to Store Byte Array in JavaScript
-
the
ArrayBuffer
Object in JavaScript - the Typed Array Views
-
Manipulating
ArrayBuffer
With Typed Array Views in JavaScript
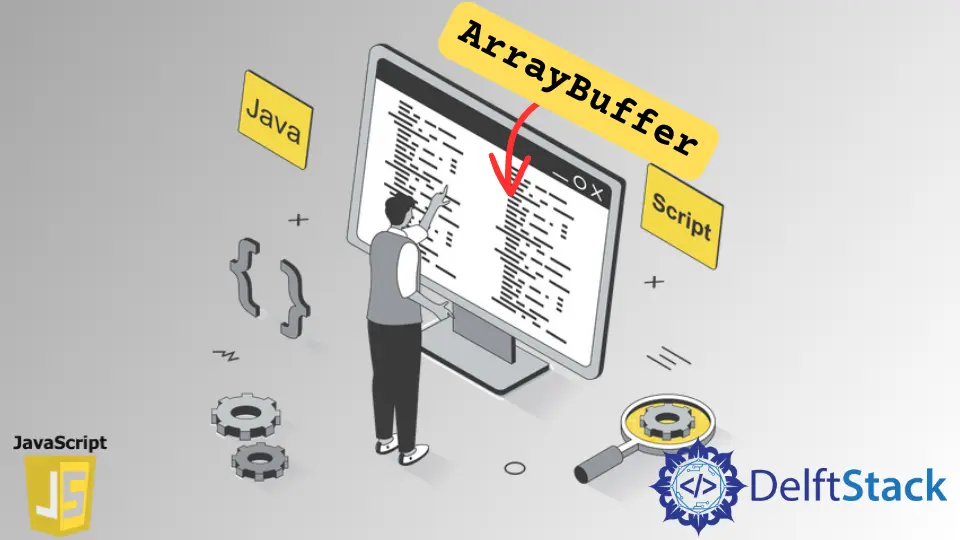
This article will discuss how to store byte arrays in JavaScript.
the ArrayBuffer
Object in JavaScript
An array of bytes or byte array is known as the ArrayBuffer
object in JavaScript. The ArrayBuffer
is not derived from JavaScript Arrays and has a fixed length in memory that can’t be changed.
In addition, you can’t directly access elements in the ArrayBuffer
object as we do in usual JavaScript arrays. It needs a separate view object to read/write.
Syntax:
new ArrayBuffer(length_in_bytes);
The above code will call the ArrayBuffer
constructor to create a new ArrayBuffer
instance with the specified byte length.
Let’s create an ArrayBuuffer
with a length of 8 bytes.
let myTypedArrayBuffer = new ArrayBuffer(8);
Ideally, the above code should create a new ArrayBuffer
with the size of 8 bytes. Now, we will check the byteLength
property of the created ArrayBuffer
object myTypedArrayBuffer
.
Code:
console.log(myTypedArrayBuffer.byteLength);
Output:
As expected, the length of the buffer is 8 bytes. It will allocate 8 bytes in the memory where each byte is assigned to zero.
This buffer doesn’t provide any mechanism to manipulate its bytes. Hence, we must use a typed array view object to read/write to/from the ArrayBuffer
.
the Typed Array Views
Typed array views are like different lenses that provide an explication of the byte array, specifically the ArrayBuffer
stored in memory. These views support numeric types such as signed and unsigned integers and floating points.
The following views have been implemented to manipulate the ArrayBuffer
object.
Type | Description |
---|---|
Uint8Array |
This view manipulates the ArrayBuffer bytes so that each byte is a separate integer. Since a byte contains 8 bits, 255 unsigned integers can be represented, and the integer range is from 0 to 255. |
Uint16Array |
This view serves ArrayBuffer as every 2 bytes in the buffer is an integer that ranges from 0 to 65535. All the integers are unsigned. |
Uint32Array |
Every 4 bytes in the ArrayBuffer is considered an integer. Hence, this view can store more than 4 billion unsigned numbers. |
Float64Array |
The Float64Array can interpret every 8 bytes in the buffer as an integer. |
As you can see, different views enable you to interpret binary data in the ArrayBuffer
as different number formats.
Manipulating ArrayBuffer
With Typed Array Views in JavaScript
All the above-mentioned typed array views follow the same constructor patterns as shown in the following.
new TypedArray(buffer); // provides and ArrayBuffer type object
new TypedArray(array_object); // provides an array-like object
new TypedArray(number_of_elements); // provides the number of integers to be in
// the typed array
new TypedArray(another_typed_array); // provides and ArrayBuffer type object
new TypedArray();
There is no actual TypedArray()
constructor in JavaScript. It will be one of the view constructors like the new Uint8Array(), new Float64Array()
.
From the previous example, let’s create a new Uint8Array
view to manipulate the already created ArrayBuffer(myTypedArrayBuffer)
.
Code:
let unsigned8BitIntView = new Uint8Array(myTypedArrayBuffer);
console.log(unsigned8BitIntView);
Output:
The Uint8Array
typed array object has been created with all the bytes filled with the value 0
.
Let’s inspect this view object’s length and actual byte length. Both should be 8
.
Code:
console.log(
'Length of the view: ' + unsigned8BitIntView.length +
'.... Byte length of the view: ' + unsigned8BitIntView.byteLength);
We have used the length
and byteLength
properties in the Uint8Array
view to obtain the above two values.
Output:
Let’s write a value to the memory buffer.
unsigned8BitIntView[0] = 200;
Now we can inspect the unsigned8BitIntView
object by iterating each element.
Code:
for (let value of unsigned8BitIntView) {
console.log(value);
}
Output:
The 200
value has been stored in the byte array. Let’s try to write 300
to the byte array.
unsigned8BitIntView[1] = 300;
We will be iterating the byte array again as in the above example.
Output:
As per the output, the integer 300
hasn’t been written to the byte array because this view can only write integers ranging from 0 to 255
.
The 300
is ignored and instead written as 44
. The following shows how the integer 44
is generated.
The integer 300
can be written as the following in binary format.
100101100
Since this view interprets 8-bit unsigned integers, the first bit will be omitted, and the remaining 8 bits will be considered.
00101100
The binary format above is integer 44
. In the same way, we can use all the typed array views to manipulate the underlying ArrayBuffer
or the array of bytes in the memory.
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript