The setInterval Loop in JavaScript
- Understanding setInterval
- Practical Use Cases for setInterval
- Best Practices for Using setInterval
- Conclusion
- FAQ
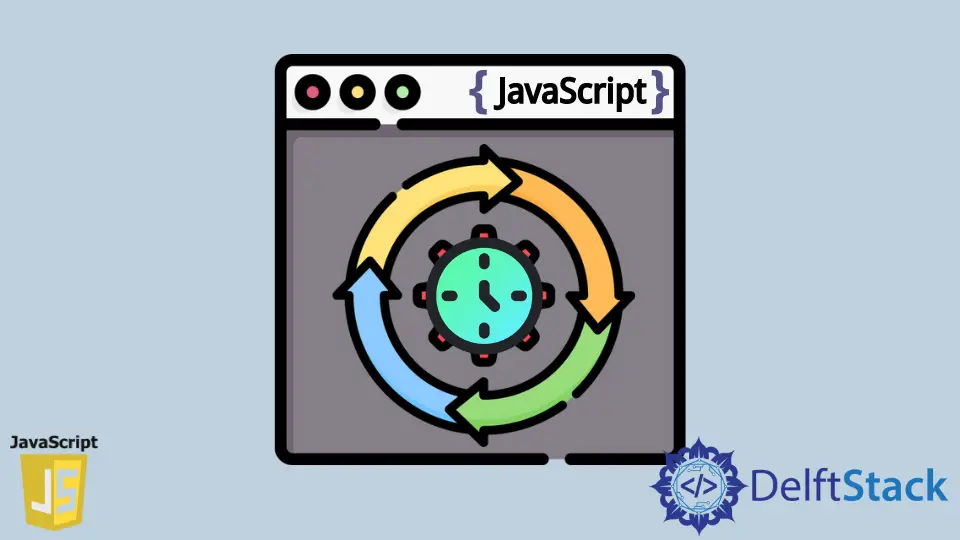
JavaScript is a powerful language that allows developers to create dynamic web applications. One of its most useful features is the setInterval
function, which enables you to execute a block of code repeatedly at specified intervals. This can be particularly handy for tasks such as updating the user interface, fetching data from a server, or creating animations.
In this article, we will delve into the setInterval
loop in JavaScript, exploring its syntax, use cases, and best practices. By the end, you will have a solid understanding of how to implement this feature effectively in your projects.
Understanding setInterval
The setInterval
function is a built-in JavaScript method that repeatedly executes a specified function at set time intervals, measured in milliseconds. The syntax is straightforward:
setInterval(function, milliseconds);
Here, function
is the code that you want to run repeatedly, and milliseconds
is the time delay between each execution. For example, if you want to update a clock every second, you would set the interval to 1000 milliseconds.
Let’s look at a simple example of setInterval
in action:
let count = 0;
const intervalId = setInterval(() => {
console.log(`Count: ${count}`);
count++;
if (count === 5) {
clearInterval(intervalId);
}
}, 1000);
Output:
Count: 0
Count: 1
Count: 2
Count: 3
Count: 4
In this example, we use setInterval
to log the count to the console every second. After five counts, we call clearInterval
to stop the execution. This is crucial to prevent the function from running indefinitely, which could lead to performance issues.
Practical Use Cases for setInterval
The setInterval
function can be used in various scenarios. Here are a few practical applications:
-
Creating Timers and Clocks: You can use
setInterval
to create countdown timers or digital clocks that update in real-time. -
Fetching Data: If you need to update your web application with new data from a server,
setInterval
can be used to make periodic AJAX calls. -
Animating Elements: For animations that require continuous updates, such as moving an object across the screen,
setInterval
can be a great tool. -
Game Loops: In game development,
setInterval
can be used to refresh the game state at regular intervals.
By understanding these use cases, you can leverage setInterval
to enhance the interactivity and responsiveness of your applications.
Best Practices for Using setInterval
While setInterval
is a powerful tool, it’s essential to use it wisely to avoid potential pitfalls. Here are some best practices to keep in mind:
-
Always Clear the Interval: As demonstrated in the previous example, always use
clearInterval
to stop the execution when it is no longer needed. This helps to prevent memory leaks and ensures that your application runs smoothly. -
Use Named Functions: Instead of using anonymous functions, consider defining a named function for your interval. This can improve readability and make it easier to manage the code.
-
Consider Performance: Be mindful of the frequency of the interval. Setting it too low can lead to performance issues, especially on resource-constrained devices.
-
Error Handling: Implement error handling within your interval function to ensure that any issues do not disrupt the execution.
-
Use with Caution: Overusing
setInterval
can lead to complicated code. If possible, consider alternatives likerequestAnimationFrame
for animations.
By following these best practices, you can effectively utilize setInterval
without running into common problems.
Conclusion
The setInterval
loop in JavaScript is a versatile function that can significantly enhance the interactivity of your web applications. Whether you’re creating a timer, fetching data, or animating elements, understanding how to use setInterval
effectively is essential for any JavaScript developer. By adhering to best practices and leveraging its capabilities, you can create smoother and more responsive user experiences. So go ahead, experiment with setInterval
, and see how it can elevate your projects.
FAQ
-
What is setInterval in JavaScript?
setInterval is a JavaScript function that repeatedly executes a specified function at defined intervals, measured in milliseconds. -
How do I stop a setInterval loop?
You can stop a setInterval loop by calling the clearInterval function with the interval ID returned by setInterval. -
Can I use setInterval for animations?
Yes, setInterval can be used for animations, but consider using requestAnimationFrame for smoother animations.
-
What happens if I don’t clear the interval?
If you don’t clear the interval, the function will continue to execute indefinitely, which can lead to performance issues. -
Is setInterval blocking?
No, setInterval is non-blocking. It allows other code to run while waiting for the next execution of the interval.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn