JavaScript Scrollable Div
-
Use the
overflow
Property to Scroll adiv
Element in JavaScript -
Use
overflowX
andoverflowY
Properties to Scroll adiv
Element in JavaScript
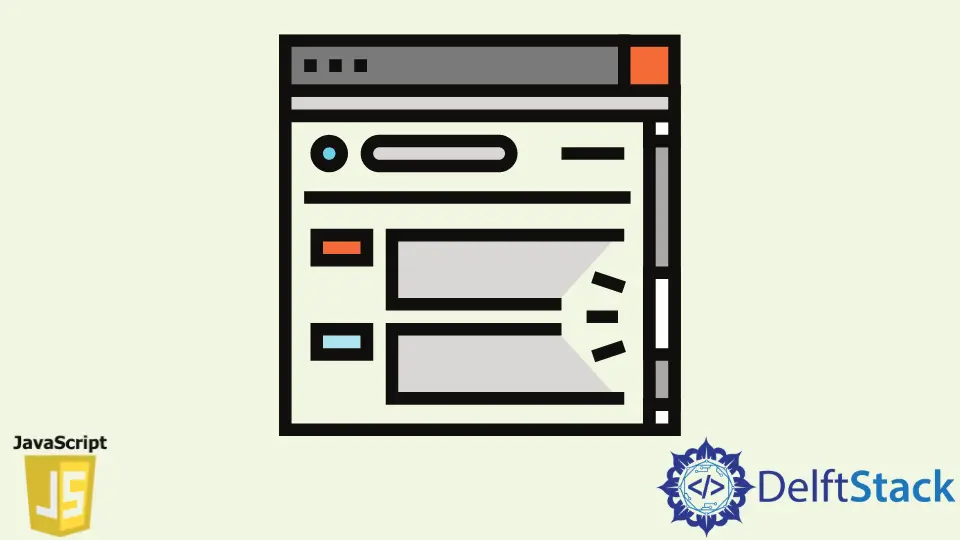
In JavaScript, we can manipulate certain events that make the interface better. Often some CSS properties’ performance can be explained by JavaScript implementation.
Just like the overflow-x
in CSS can do the same task in JavaScript as overflowX
.
Our task is to make a div
element scrollable. We will not be focusing on the offsetHeight/Width
or the basic height width of the content; rather, we will set a div with a static size.
But the content can be of variable length. We will see two examples that will cover the usage of overflow
and overflowX and overflowY
.
Let’s check the codes.
Use the overflow
Property to Scroll a div
Element in JavaScript
The overflow
property for the value auto
will automatically create a vertical scrollbar if the content is larger than the div
size. This one property will solve the case of making a scrollable div
element.
In the following code lines, a demo is presented.
Code Snippet:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>test</title>
<style>
div{
background: powderblue;
}
#scroll{
height:100px;
width: 200px;
}
</style>
</head>
<body>
<div id="scroll">
<p>Lorem ipsum dolor sit amet, consectetur adipisicing elit. Perferendis reprehenderit earum, rem tenetur quaerat, ab, nostrum ducimus totam quis natus placeat eos vitae? Sint eos, ab eum repellendus ex praesentium.</p>
</div>
<script>
document.getElementById('scroll').style.overflow = 'auto';
</script>
</body>
</html>
Output:
Use overflowX
and overflowY
Properties to Scroll a div
Element in JavaScript
We set the x-axis
scrollbar to none
according to these property sets. And thus, the vertical scrollbar is set with the value of overflowY
to auto
.
Consequently, when the content gets a larger size than the div
, the scrollbar appears and functions. The code fence gives a better preview.
Code Snippet:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>JS Bin</title>
<style>
div{
background: lavender;
}
#scroll{
height:100px;
width: 200px;
}
</style>
</head>
<body>
<div id="scroll">
<p>Lorem ipsum dolor sit amet, consectetur adipisicing elit. Perferendis reprehenderit earum, rem tenetur quaerat, ab, nostrum ducimus totam quis natus placeat eos vitae? Sint eos, ab eum repellendus ex praesentium.</p>
</div>
<script>
document.getElementById('scroll').style.overflowX='none';
document.getElementById('scroll').style.overflowY='auto';
</script>
</body>
</html>
Output: