How to Scroll to Top in JavaScript
- Method 1: Using window.scrollTo()
- Method 2: Smooth Scrolling with window.scrollTo()
- Method 3: Using jQuery for Scroll to Top
- Method 4: CSS Scroll Snap
- Conclusion
- FAQ
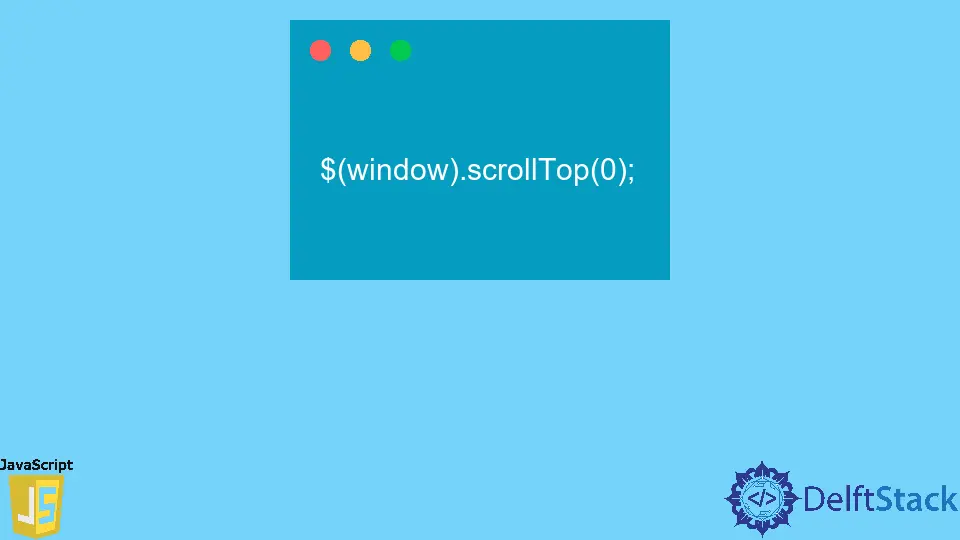
Scrolling to the top of a webpage is a common interaction that enhances user experience. Whether it’s after reading a long article or exploring a product page, users often appreciate a quick way to return to the top.
In this tutorial, we’ll explore various methods to achieve this using JavaScript. From simple functions to smooth scrolling effects, you will learn how to implement these techniques effectively. This guide is designed for developers of all levels, so whether you’re a beginner or an experienced coder, you’ll find valuable insights here. Let’s dive into the world of JavaScript scrolling!
Method 1: Using window.scrollTo()
The window.scrollTo()
method is one of the simplest ways to scroll to the top of a page. This method allows you to specify the x and y coordinates where you want the page to scroll. To scroll to the top, you can set both coordinates to zero. Here’s a basic example of how to implement this:
function scrollToTop() {
window.scrollTo(0, 0);
}
document.getElementById("scrollButton").addEventListener("click", scrollToTop);
In this code snippet, we define a function called scrollToTop()
. When invoked, it uses window.scrollTo(0, 0)
to scroll the page to the top. We also add an event listener to a button with the ID scrollButton
, so when the button is clicked, the function is executed.
This method is straightforward and effective for immediate scrolling. However, it does not provide a smooth transition, which can be less visually appealing. For a better user experience, you can enhance this method with smooth scrolling using CSS.
Method 2: Smooth Scrolling with window.scrollTo()
To create a smoother scrolling effect, you can use the behavior
property of the window.scrollTo()
method. By setting the behavior to smooth
, the scrolling action becomes more visually appealing. Here’s how you can modify the previous example:
function scrollToTop() {
window.scrollTo({
top: 0,
behavior: 'smooth'
});
}
document.getElementById("scrollButton").addEventListener("click", scrollToTop);
In this updated code, we pass an object to the window.scrollTo()
method, specifying the top
property as 0
and the behavior
property as smooth
. This creates a smooth scrolling effect when the button is clicked.
This method is particularly useful for websites with long content, as it enhances the user’s navigation experience. Smooth scrolling adds a touch of elegance to your web application and keeps users engaged.
Method 3: Using jQuery for Scroll to Top
If you’re using jQuery in your project, you can take advantage of its built-in methods to simplify the scrolling process. jQuery provides a convenient way to animate the scroll action. Here’s how you can implement it:
$("#scrollButton").click(function() {
$("html, body").animate({ scrollTop: 0 }, 800);
});
In this example, we use jQuery’s animate()
method to scroll the page to the top. The scrollTop: 0
property indicates that we want to scroll to the top, and the 800
specifies the duration of the animation in milliseconds.
Using jQuery makes it easy to create smooth animations with minimal code. If your project already includes jQuery, this method is an excellent choice for adding a scroll-to-top feature without much overhead.
Method 4: CSS Scroll Snap
For modern browsers, you can also leverage CSS Scroll Snap to create a smooth scrolling effect. While this method doesn’t directly scroll to the top, it allows you to create a snapping effect that can enhance the overall scrolling experience. Here’s how you can implement it:
html {
scroll-behavior: smooth;
}
section {
height: 100vh;
scroll-snap-align: start;
}
In this CSS snippet, we set scroll-behavior: smooth
on the html
element, which enables smooth scrolling for the entire page. Each section
has a height of 100vh
, and the scroll-snap-align: start
property ensures that the sections snap into place as you scroll.
While this method doesn’t provide a direct button to scroll to the top, it enhances the user experience by making scrolling feel more fluid. This is particularly useful for single-page applications or long scrolling websites.
Conclusion
In this tutorial, we’ve explored various methods to scroll to the top of a webpage using JavaScript. From the straightforward window.scrollTo()
method to the elegant jQuery animations and modern CSS techniques, you have a range of options to choose from. Each method has its own advantages, and the best choice depends on your specific needs and project requirements. By implementing these techniques, you’ll enhance user experience and make your web applications more interactive. Happy coding!
FAQ
-
how do i implement a scroll to top button?
You can create a button and add an event listener that triggers the scroll function when clicked. Usewindow.scrollTo()
for immediate scrolling or jQuery’sanimate()
for smooth scrolling. -
can i customize the scroll speed?
Yes, if you’re using jQuery, you can specify the duration of the scroll animation in milliseconds. For smooth scrolling with CSS, you can adjust the duration through CSS transitions. -
is smooth scrolling supported in all browsers?
Most modern browsers support smooth scrolling through CSS. However, older browsers may not support this feature. Always check compatibility if targeting a wide audience. -
can i use scroll to top in a single-page application?
Absolutely! Scroll to top functionality is particularly useful in single-page applications where users navigate through various sections without reloading the page. -
what is the best method for scrolling to the top?
The best method depends on your project needs. If you want a quick solution, usewindow.scrollTo()
. For a more polished user experience, consider using jQuery or CSS smooth scrolling.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn