How to Round a Number to 2 Decimal Places in JavaScript
-
Use the
.toFixed()
Method to Round a Number To 2 Decimal Places in JavaScript -
Use the
Math.round()
Function to Round a Number To 2 Decimal Places in JavaScript - Use Double Rounding to Round a Number To 2 Decimal Places in JavaScript
- Using the Custom Function to Round a Number To 2 Decimal Places in JavaScript
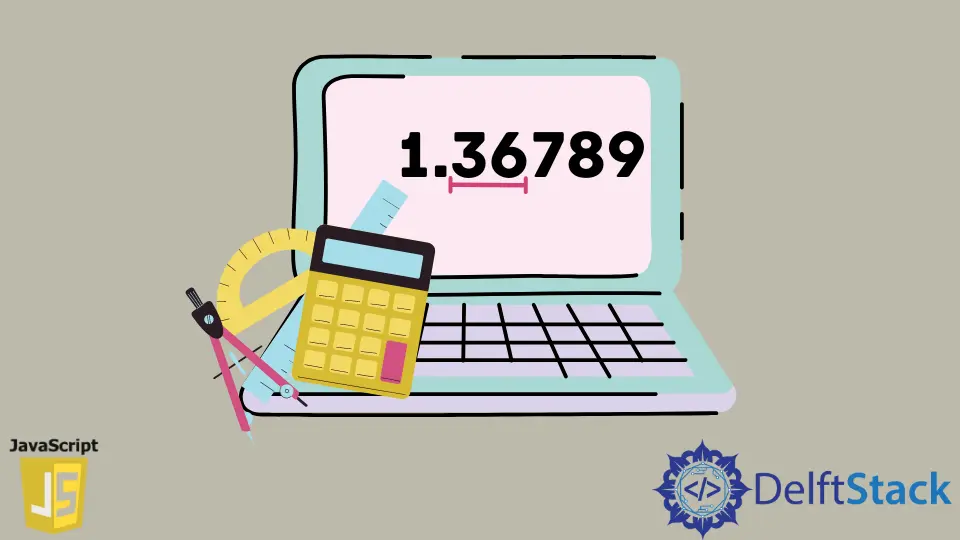
This tutorial introduces how to round a number to 2 decimal places in JavaScript.
Use the .toFixed()
Method to Round a Number To 2 Decimal Places in JavaScript
We apply the .toFixed()
method on the number and pass the number of digits after the decimal as the argument.
var numb = 12312214.124124124;
numb = numb.toFixed(2);
This method does not yield accurate results in some cases, and there are better methods than this. If a number rounds of 1.2
, then it will show 1.20
. If a number like 2.005
is given, it will return 2.000
instead of 2.01
.
Use the Math.round()
Function to Round a Number To 2 Decimal Places in JavaScript
We take the number and add a very small number, Number.EPSILON
, to ensure the number’s accurate rounding. We then multiply by number with 100
before rounding to extract only the two digits after the decimal place. Finally, we divide the number by 100 to get a max of 2 decimal places.
var numb = 212421434.533423131231;
var rounded = Math.round((numb + Number.EPSILON) * 100) / 100;
console.log(rounded);
Output:
212421434.53
Although This method improves over .toFixed()
, it is still not the best solution and will also not round of 1.005
correctly.
Use Double Rounding to Round a Number To 2 Decimal Places in JavaScript
In this method, we use the .toPrecision()
method to strip off the floating-point rounding errors introduced during the intermediate calculations in single rounding.
function round(num) {
var m = Number((Math.abs(num) * 100).toPrecision(15));
return Math.round(m) / 100 * Math.sign(num);
}
console.log(round(1.005));
Output:
1.01
Using the Custom Function to Round a Number To 2 Decimal Places in JavaScript
function roundToTwo(num) {
return +(Math.round(num + 'e+2') + 'e-2');
}
console.log(roundToTwo(2.005));
This custom function takes care of all the corner cases, and rounding of decimals like 1.005
is handled well by this function.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn