JavaScript Right Click Menu
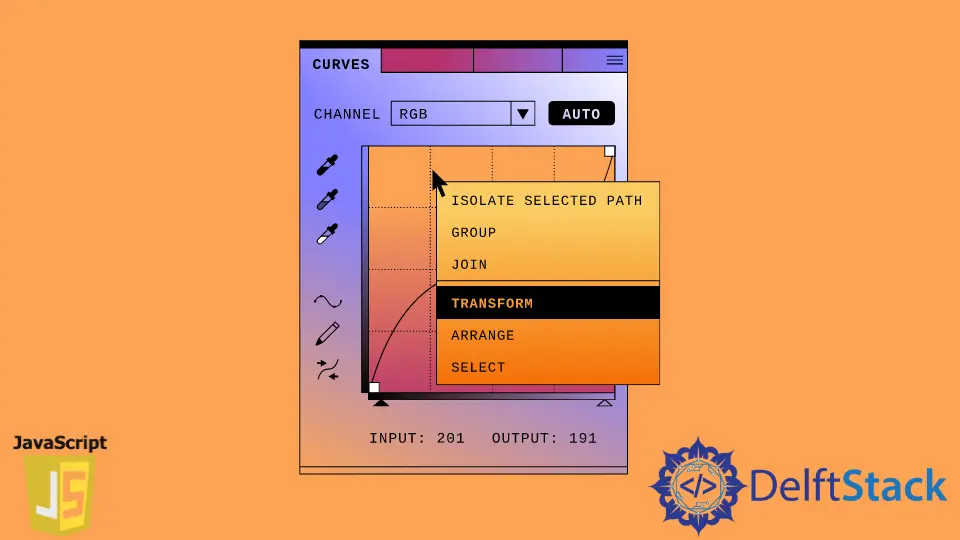
The context menu is a convention that allows us to create a custom right-click menu.
A right-click menu is a simple panel previewed when clicking the mouse right button. These options of the menu can have different functions to play.
Use the oncontextmenu
Event to Enable JavaScript Right-Click Menu
The oncontextmenu
event will enable the creation of the div
element dynamically every time the right click is ensured.
We will set a style for the menu positioning. If we do not set the event-derived positions, the menu will be static on the part of the page.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<title>Test</title>
<style>
#contextMenu {
position: fixed;
background:teal;
color: white;
cursor: pointer;
border: 1px black solid
}
#contextMenu > p {
padding: 0 1rem;
margin: 0
}
#contextMenu > p:hover {
background: black;
color: white;
}
</style>
</head>
<body>
<script>
oncontextmenu = (e) => {
e.preventDefault()
var menu = document.createElement("div")
menu.id = "contextMenu"
menu.style = `top:${e.pageY-10}px;left:${e.pageX-40}px`
menu.onmouseleave = () => contextMenu.outerHTML = ''
menu.innerHTML = "<p onclick='alert(`1 It is!`)'>Choose 1</p><p onclick='alert(`2 It is!`)'>Choose 2</p><p onclick='alert(`3 It is!`)'>Choose 3</p><p onclick='alert(`Thank you!`)'>No thanks</p>"
document.body.appendChild(menu)
}
</script>
</body>
</html>
Output:
Inside the oncontextmenu
event, we set the onmouseleave
event to enable the option box to vanish if the cursor is removed from the menu.
We will get the desired output in the windows alert on choosing one option. This is how to make a context menu on right-click.