RGB Color Model in JavaScript
- What is the RGB Color Model?
- Creating Colors Using RGB in JavaScript
- Converting Hex to RGB in JavaScript
- Adjusting Colors with RGB in JavaScript
- Conclusion
- FAQ
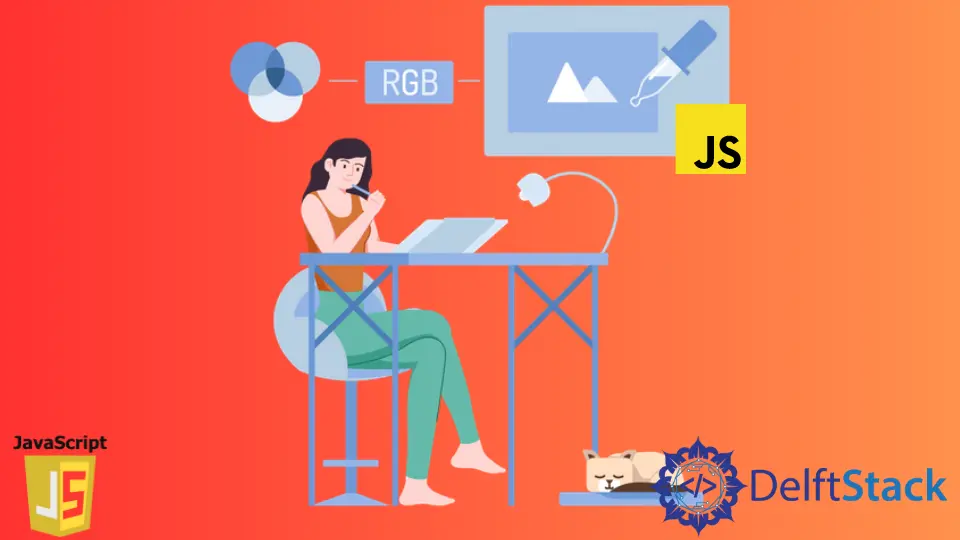
In today’s post, we are going to learn about the RGB color model in JavaScript. The RGB color model is a foundational concept in web development and design, allowing developers to create visually appealing interfaces. RGB stands for Red, Green, and Blue, which are the primary colors of light. By combining these colors in various intensities, you can produce a wide spectrum of colors.
This article will guide you through the basics of the RGB color model, how to implement it in JavaScript, and some practical examples to help you better understand its application. Whether you’re a beginner or an experienced developer, this guide will enhance your knowledge and skills in working with colors in JavaScript.
What is the RGB Color Model?
The RGB color model is a method of representing colors through the combination of red, green, and blue light. Each color is represented by a triplet of values, where each value ranges from 0 to 255. A value of 0 means no intensity of that color, while 255 represents full intensity. For example, the color white is represented as (255, 255, 255), as it contains the maximum intensity of all three colors. Conversely, black is (0, 0, 0), indicating no light at all.
The RGB model is widely used in digital displays because it aligns with how screens emit light. This model is particularly useful in web development, where colors are often defined in CSS or manipulated through JavaScript. Understanding the RGB model allows developers to create dynamic and interactive web applications that are visually engaging.
Creating Colors Using RGB in JavaScript
To create colors using the RGB model in JavaScript, you can use the rgb()
CSS function. This function takes three parameters corresponding to the red, green, and blue components of the color. Below is an example of how to create a color using RGB values in JavaScript:
const red = 255;
const green = 0;
const blue = 0;
const rgbColor = `rgb(${red}, ${green}, ${blue})`;
document.body.style.backgroundColor = rgbColor;
Output:
The background color of the webpage will be set to red.
In this code snippet, we define three variables for red, green, and blue. We then create a string that represents the RGB color using template literals. Finally, we apply this color to the background of the webpage by setting the backgroundColor
property of the document.body
object. This simple example showcases how you can manipulate colors dynamically using JavaScript.
Converting Hex to RGB in JavaScript
Sometimes, you may encounter colors represented in hexadecimal format (hex). To convert a hex color code to RGB, you can create a function that performs the conversion. Here’s how you can do it in JavaScript:
function hexToRgb(hex) {
let r = parseInt(hex.slice(1, 3), 16);
let g = parseInt(hex.slice(3, 5), 16);
let b = parseInt(hex.slice(5, 7), 16);
return `rgb(${r}, ${g}, ${b})`;
}
const hexColor = "#ff5733";
const rgbColor = hexToRgb(hexColor);
console.log(rgbColor);
Output:
rgb(255, 87, 51)
In this example, the hexToRgb
function takes a hex color string as input. It extracts the red, green, and blue components using the slice
method and converts them from hexadecimal to decimal using parseInt
. The function then returns the corresponding RGB string. When we run this code with the hex color #ff5733
, it converts it to its RGB equivalent, which is rgb(255, 87, 51)
.
Adjusting Colors with RGB in JavaScript
Another interesting aspect of working with the RGB color model in JavaScript is the ability to adjust colors programmatically. You can create functions that lighten or darken a color based on its RGB values. Here’s an example of how you can lighten an RGB color:
function lightenColor(rgb, percent) {
let [r, g, b] = rgb.match(/\d+/g).map(Number);
r = Math.min(255, Math.floor(r + (r * percent)));
g = Math.min(255, Math.floor(g + (g * percent)));
b = Math.min(255, Math.floor(b + (b * percent)));
return `rgb(${r}, ${g}, ${b})`;
}
const originalColor = "rgb(100, 150, 200)";
const lightenedColor = lightenColor(originalColor, 0.2);
console.log(lightenedColor);
Output:
rgb(120, 180, 240)
In this code snippet, the lightenColor
function takes an RGB color string and a percentage as parameters. It uses a regular expression to extract the RGB values and converts them to numbers. The function then calculates the new RGB values by adding a percentage of the original value. Finally, it returns the new lightened color. When we apply this function to the original color rgb(100, 150, 200)
with a 20% lightening effect, we get rgb(120, 180, 240)
.
Conclusion
Understanding the RGB color model in JavaScript is essential for any web developer. This model allows for the creation and manipulation of colors in a way that enhances the user experience. Through practical examples, we explored how to create colors, convert hex values to RGB, and even adjust colors programmatically. By mastering these techniques, you can take your web applications to the next level, making them not only functional but also visually appealing. Whether you’re designing a simple webpage or a complex application, the RGB color model is a powerful tool in your development arsenal.
FAQ
-
What is the RGB color model?
The RGB color model is a way to represent colors using red, green, and blue light, where each color is defined by a triplet of values ranging from 0 to 255. -
How do I create an RGB color in JavaScript?
You can create an RGB color in JavaScript using thergb()
CSS function and setting it to a style property, likedocument.body.style.backgroundColor
. -
Can I convert hex colors to RGB in JavaScript?
Yes, you can convert hex colors to RGB using a custom function that extracts the red, green, and blue components from the hex string. -
How can I lighten a color in JavaScript?
You can lighten a color by increasing its RGB values by a certain percentage, ensuring that the values do not exceed 255. -
Why is the RGB model important in web development?
The RGB model is crucial in web development as it allows developers to create visually appealing designs and user interfaces by manipulating colors effectively.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn