How to Reverse Array in JavaScript
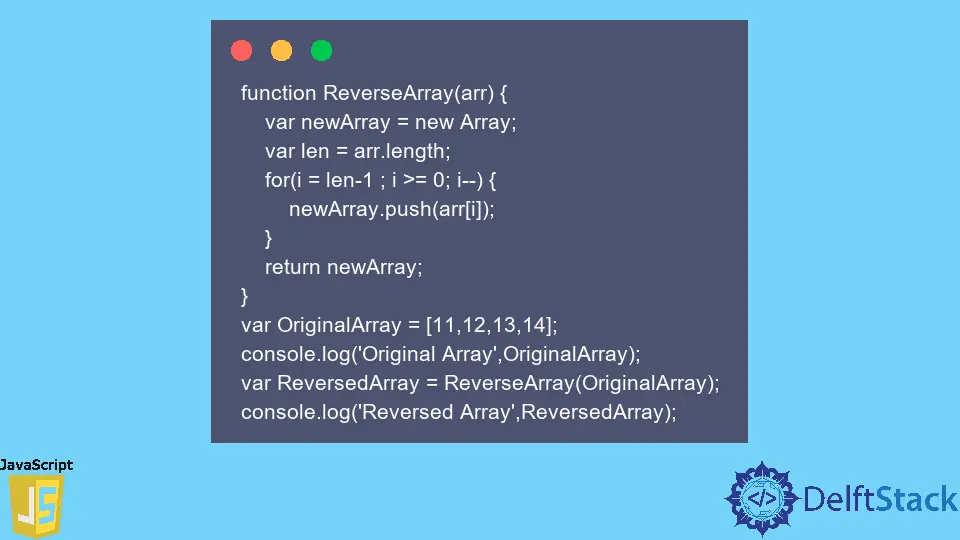
Reversing an array in JavaScript is a common task that can be accomplished in various ways. Whether you’re a beginner or an experienced developer, understanding how to manipulate arrays is crucial for effective programming.
In this article, we will explore two primary methods to reverse an array in JavaScript: using the built-in reverse()
function and creating a custom function from scratch. Both methods have their unique advantages and can be applied in different scenarios. By the end of this article, you’ll have a clear understanding of how to reverse arrays and when to use each method, enriching your JavaScript skill set.
Method 1: Using the reverse() Function
JavaScript provides a built-in method called reverse()
that allows you to reverse the elements of an array in place. This means the original array is modified, and the elements are rearranged in the opposite order. The reverse()
function is straightforward to use and is one of the most efficient ways to reverse an array.
Here’s how you can use the reverse()
method:
let array = [1, 2, 3, 4, 5];
array.reverse();
console.log(array);
Output:
[5, 4, 3, 2, 1]
In this example, we start with an array containing numbers from 1 to 5. By calling the reverse()
method on the array, we modify it directly. The original array is now reversed, and when we log it to the console, we see that the order of elements has changed from [1, 2, 3, 4, 5]
to [5, 4, 3, 2, 1]
. This method is particularly useful when you want a quick and efficient way to reverse an array without creating a new one.
Method 2: Creating Your Own Reverse Function
While the reverse()
method is convenient, there may be situations where you want to implement your own custom function to reverse an array. This can be a great exercise for understanding array manipulation and can also be useful in scenarios where you want more control over the reversing process.
Here’s a simple implementation of a custom reverse function:
function customReverse(arr) {
let reversedArray = [];
for (let i = arr.length - 1; i >= 0; i--) {
reversedArray.push(arr[i]);
}
return reversedArray;
}
let myArray = [1, 2, 3, 4, 5];
let reversed = customReverse(myArray);
console.log(reversed);
Output:
[5, 4, 3, 2, 1]
In this code, we define a function called customReverse
that takes an array as an argument. We create an empty array called reversedArray
to store the elements in reverse order. Using a for
loop, we iterate through the input array from the last index to the first. During each iteration, we push the current element onto the reversedArray
. Finally, we return the newly created array. When we call this function with myArray
, we get the reversed array as expected.
Creating your own function gives you the flexibility to modify the logic as needed and can be a valuable learning experience.
Conclusion
Reversing an array in JavaScript can be accomplished easily with the built-in reverse()
method or by creating a custom function. The built-in method is efficient and straightforward, while crafting your own function allows for greater control and understanding of array manipulation. Whether you choose to use the built-in method or create your own, mastering these techniques will enhance your JavaScript programming skills and help you tackle more complex coding challenges with confidence.
FAQ
-
What does the
reverse()
function do in JavaScript?
Thereverse()
function reverses the elements of an array in place, modifying the original array. -
Can I reverse an array without modifying the original array?
Yes, you can create a custom function that returns a new reversed array without altering the original. -
Is the
reverse()
method efficient for large arrays?
Thereverse()
method is generally efficient, but performance can vary based on the size of the array and the JavaScript engine. -
Can I reverse an array of strings using these methods?
Absolutely! Both methods can be applied to arrays containing strings or any other data type. -
What are some other array methods I should know in JavaScript?
Some other useful array methods includemap()
,filter()
,reduce()
, andforEach()
, each serving different purposes in array manipulation.
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript