How to Remove Element by Id in JavaScript
-
Set the
outerHTML
Attribute to Empty String to Remove Element by Id in JavaScript -
Use
removeChild()
to Remove Element by Id in JavaScript -
Use
remove()
to Remove Element by Id in JavaScript
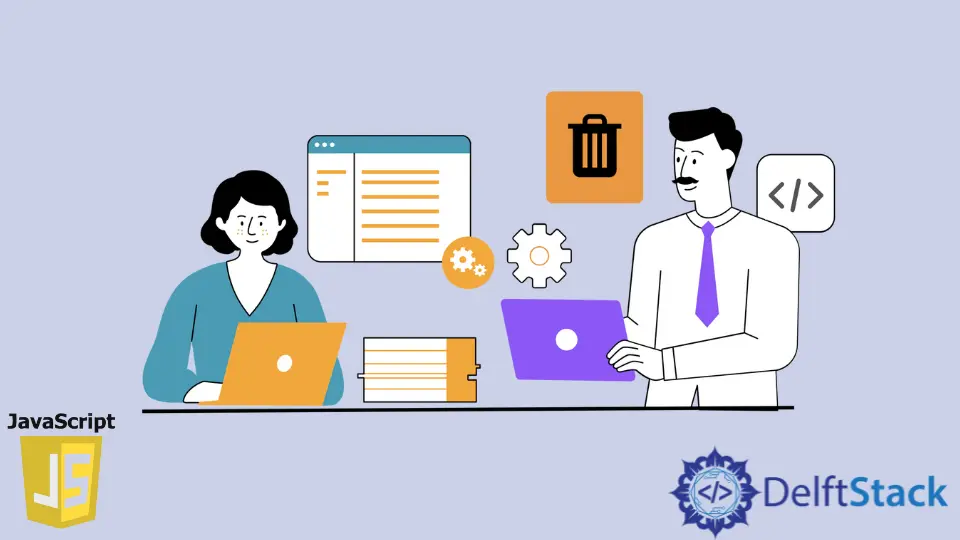
This tutorial introduces how to remove an HTML element using its id in JavaScript.
Set the outerHTML
Attribute to Empty String to Remove Element by Id in JavaScript
In this method, we select the element and erase the HTML content by assigning an empty string.
<!DOCTYPE html>
<html lang="en">
<head>
<title>Document</title>
</head>
<body>
<h1 id="app">Hello World!!</h1>
<p id="removeme">Remove Me</p>
</body>
<script>
document.getElementById("removeme").outerHTML = "";
</script>
</html>
It’s a simple method, but it’s rarely used due to the following limitations:
- It is slower by 5-10% in speed than
removeChild()
and other newer ES5 methods likeremove()
. - It is not supported in older versions of Internet Explorer.
Use removeChild()
to Remove Element by Id in JavaScript
We first select the element using its id and then call its parent’s removeChild()
function in this method. This method provides the advantage of maintaining the tree structure of DOM.
It forces us to mimic the internal behavior of removing an element from the tree so that we don’t delete the parent node itself.
<!DOCTYPE html>
<html lang="en">
<head>
<title>Document</title>
</head>
<body>
<h1 id="app">Hello World!!</h1>
<p id="removeme">Remove Me</p>
</body>
<script>
function remove(id)
{
var element = document.getElementById(id);
return element.parentNode.removeChild(element);
}
remove("removeme");
</script>
</html>
Use remove()
to Remove Element by Id in JavaScript
The remove()
method was introduced as part of ES5. It allows us to remove the element directly without going to its parent. But unlike the removeChild()
method, it doesn’t return a reference to the removed node.
<!DOCTYPE html>
<html lang="en">
<head>
<title>Document</title>
</head>
<body>
<h1 id="app">Hello World!!</h1>
<p id="removeme">Remove Me</p>
</body>
<script>
document.getElementById("removeme").remove();
</script>
</html>
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn