How to Remove a Character From a String in JavaScript
-
Use the
replace()
Method With Regular Expression in JavaScript - Remove a Specified Character at the Given Index in JavaScript
- Remove First Instance of Character in a String in JavaScript
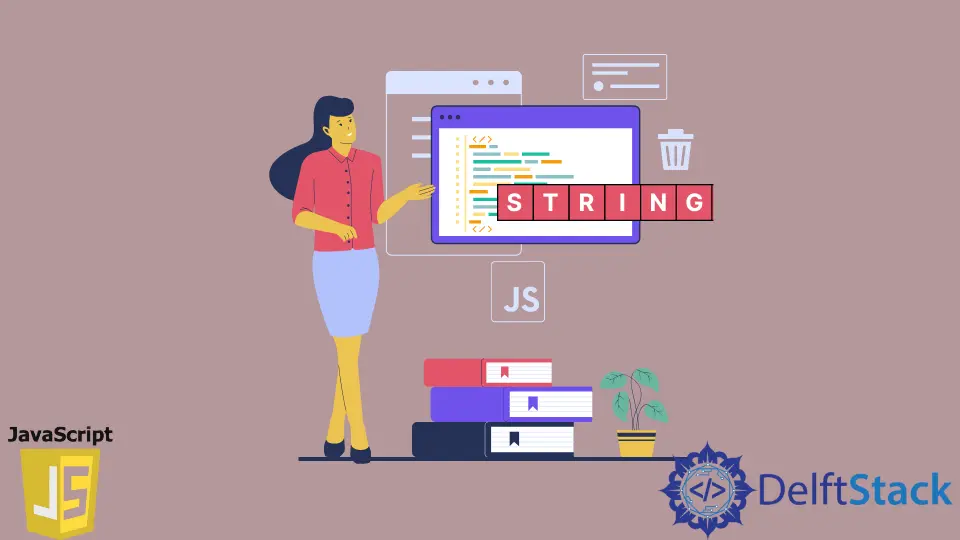
JavaScript has different methods to remove a specific character from a string. We will introduce how to remove a character from a string in JavaScript.
Use the replace()
Method With Regular Expression in JavaScript
We use the replace()
method with the regular expression to remove all instances of the specified character in a string in JavaScript.
JavaScript replace()
Regular Expression Syntax
replace(/regExp/g, '');
Example:
<!DOCTYPE html>
<html>
<head>
<title>
How to remove all instances of the specified character in a string?
</title>
</head>
<body>
<h1>
DelftStack
</h1>
<b>
How to remove all instances of the specified character in a string?
</b>
<p>The original string is DelftStack</p>
<p>
New Output is:
<span id="outputWord"></span>
</p>
<button onclick="removeCharacterFromString()">
Remove Character
</button>
<script type="text/javascript">
const removeCharacterFromString = () => {
originalWord = 'DelftStack';
newWord = originalWord.replace(/t/g, '');
document.querySelector('#outputWord').textContent
= newWord;
}
</script>
</body>
</html>
Output:
The original string is DelftStack
New Output is: DelfSack
Remove a Specified Character at the Given Index in JavaScript
When we need to remove a character when we have more than one instance of this character in a string, for example, remove the character t
from a string DelftStack
, we can use the slice()
method to get two strings before and after the given index and concatenate them.
Example:
<!DOCTYPE html>
<html>
<head>
<title>
How to remove Specified Character at a Given Index in a string?
</title>
</head>
<body>
<h1>
DelftStack
</h1>
<b>
How to remove Specified Character at a Given Index in a string?
</b>
<p>The original string is DelftStack</p>
<p>
New Output is:
<span id="outputWord"></span>
</p>
<button onclick="removeCharacterFromString(5)">
Remove Character
</button>
<script type="text/javascript">
const removeCharacterFromString = (position) => {
originalWord = 'DelftStack';
newWord = originalWord.slice(0, position - 1)
+ originalWord.slice(position, originalWord.length);
document.querySelector('#outputWord').textContent
= newWord;
}
</script>
</body>
</html>
Remove First Instance of Character in a String in JavaScript
We can use the replace()
method without a regular expression to remove only the first instance of a character from a string in JavaScript. We pass the character to be removed as the first argument and the empty string ''
as the second argument.
Example:
<!DOCTYPE html>
<html>
<head>
<title>
How to remove First Instance of Character in a string?
</title>
</head>
<body>
<h1>
DelftStack
</h1>
<b>
How to remove First Instance of Character in a string?
</b>
<p>The original string is DelftStack</p>
<p>
New Output is:
<span id="outputWord"></span>
</p>
<button onclick="removeCharacterFromString()">
Remove Character
</button>
<script type="text/javascript">
const removeCharacterFromString = () => {
originalWord = 'DelftStack';
newWord = originalWord.replace('t', '');
document.querySelector('#outputWord').textContent
= newWord;
}
</script>
</body>
</html>