JavaScript DOM Ready
-
Use the jQuery
ready()
Function to Call Functions When DOM Is Ready - Vanilla JavaScript Approach to Call Functions When DOM Is Ready
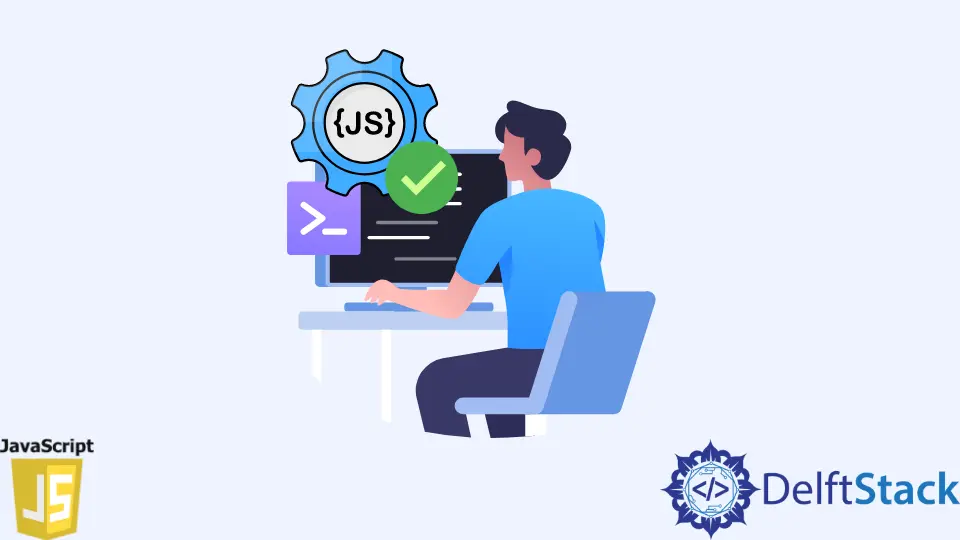
The loading of a website starts from downloading the HTML document. Once the HTML doc is downloaded, the HTML is parsed, and required assets are downloaded. After the HTML is parsed, the DOM and CSS are rendered in parallel. After this, the browser takes care of the JavaScript onload
functions. This is the timeframe we want to call functions because HTML, CSS, DOM are correctly rendered. This tutorial teaches how to call a function when the DOM is ready.
Use the jQuery ready()
Function to Call Functions When DOM Is Ready
The ready()
function in jQuery executes the code when the DOM is ready. It waits for all the elements to be fully loaded and then tries to access and manipulate them. We can select the document
element, an empty element to call a function, or even directly call a function.
$(document).ready(function() {
// callback function / handler
});
It is a full cross-browser compliant method and can be called from anywhere in the HTML code.
Vanilla JavaScript Approach to Call Functions When DOM Is Ready
We can create the JavaScript equivalent of jQuery’s ready()
function by using the DOMContentLoaded
event. We can add an event listener for DOMContentLoaded
and call our desired function with that event listener. But there is one catch, the callback will not be triggered if the event has already occurred. So, we take care of this condition by checking the ready state of the document object.
const callback = function() {};
if (document.readyState === 'complete' ||
document.readyState === 'interactive') {
setTimeout(callback, 1);
} else {
document.addEventListener('DOMContentLoaded', callback);
}
There are few tricks other than these methods that help us to solve this problem but may not be ideal in all cases.
- Use the window’s
onload
attribute to call the JavaScript function. - Use the body’s
onload
attribute to call the JavaScript function. This method can be terribly slow as it waits for every single element to be loaded. - We can call the function to be executed from the end of the body. At the end of the body, DOM is appropriately rendered and ready. So, there are no issues with function calls related to any element. It works much faster than the body
onload
handler because it does not wait for the images to be loaded completely.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn