How to Generate Random String in JavaScript
Kirill Ibrahim
Feb 02, 2024
JavaScript
JavaScript String
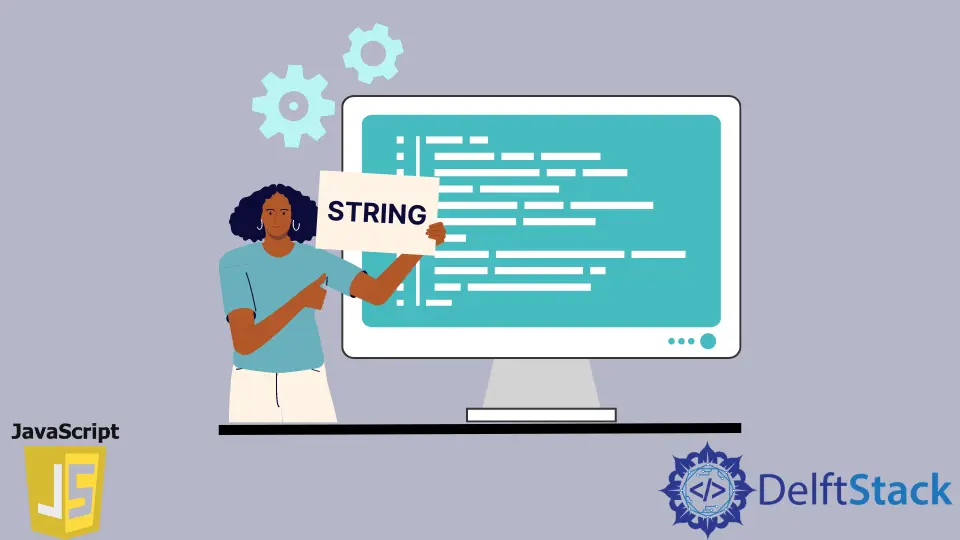
This article will introduce multiple ways to generate random strings in JavaScript. Every method below will have a code example, which you can run on your machine.
Use the for
Loop to Generate Random Strings
We use the Math.random()
method to generate the random number between 0 and 1.
Math.floor(Math.random() * charactersLength)
will generate a random number between 0 and the length of the declared array.
We use the for
loop to create the required length of the random string. A random character is generated in each iteration.
Example:
const generateRandomString =
(num) => {
const characters =
'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789';
let result1 = ' ';
const charactersLength = characters.length;
for (let i = 0; i < num; i++) {
result1 +=
characters.charAt(Math.floor(Math.random() * charactersLength));
}
return result1;
}
const displayRandomString =
() => {
let randomStringContainer = document.getElementById('random_string');
randomStringContainer.innerHTML = generateRandomString(8);
}
console.log(generateRandomString(5));
Use Built-In Methods to Generate Random Strings
We can use the following built-in functions to generate random string:
- The
Math.random()
method to generate random characters. - The
toString(36)
method representing[a-zA-Z0-9]
. Read more abouttoString()
. - The
substring(startIndex, endIndex)
method to return the specified number of characters.
Example
const generateRandomString =
(num) => {
const characters =
'ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz0123456789';
let result1 = Math.random().toString(36).substring(0, num);
return result1;
}
console.log(generateRandomString(7));
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe