How to Generate Random Number in a Specified Range in JavaScript
-
Math.random()
Function - Algorithm to Generate Random Number in a Specified Range in JavaScript
- Example Code
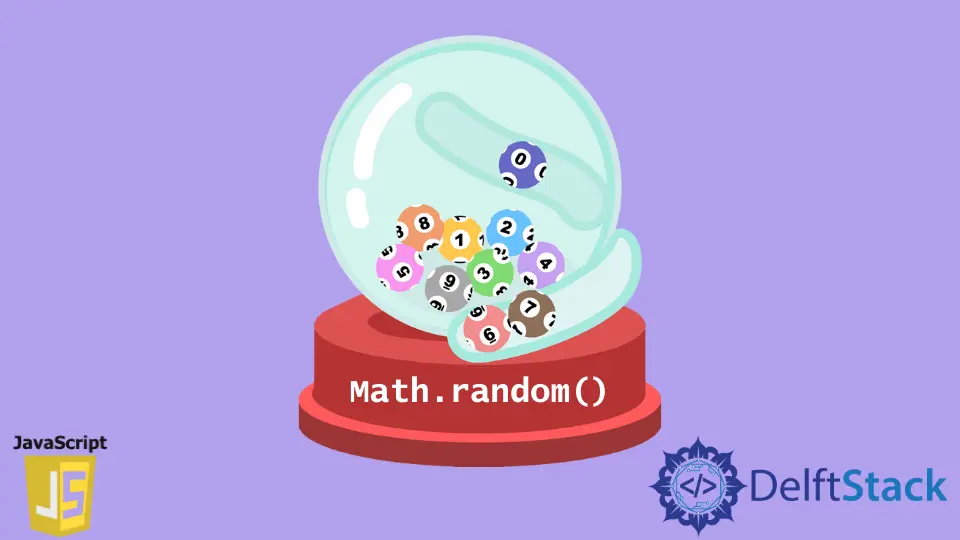
This tutorial introduces how to generate random numbers inside a specified range in JavaScript. We will use the Math.random()
function to generate random numbers and then shift them to a number inside the specified range.
Math.random()
Function
This function returns a floating-point, pseudo-random number in the range 0
(inclusive) to 1
(exclusive) with an approximately uniform distribution over that range. But it doesn’t return cryptographically secure numbers.
Algorithm to Generate Random Number in a Specified Range in JavaScript
{{ % step %}}
-
Generate a number between the range
0
and1
and store it in a variable namedrand
. -
Calculate the total range
range
asmax-min+1
. -
Multiply
range
byrand
and add it tomin
to get the random number inside the specified range.
{{ % /step %}}
Example Code
function getRandomIntInclusive(min, max) {
min = Math.ceil(min);
max = Math.floor(max);
return Math.floor(Math.random() * (max - min + 1) + min);
}
getRandomIntInclusive(2, 5);
Output:
4
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedInRelated Article - JavaScript Math
- How to Convert Radians to Degrees in JavaScript
- BigDecimal in JavaScript
- How to Add Two Numbers in JavaScript
- How to Use Scientific Notation in JavaScript
- How to Calculate the Percentage in JavaScript
- How to Round Number to the Nearest 10 in JavaScript