JavaScript Window Print Page Button
- Understanding window.print()
- Creating a Print Button in HTML
- Customizing Print Styles with CSS
- Best Practices for Using window.print()
- Conclusion
- FAQ
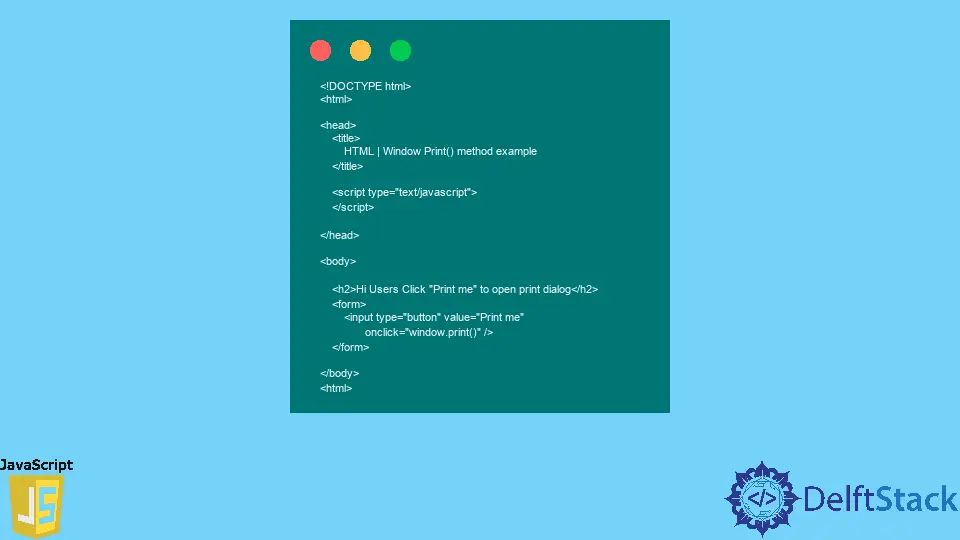
Printing directly from a web page is a common requirement for many users and developers alike. Fortunately, JavaScript provides a straightforward method to achieve this with the window.print()
function. This simple function opens a print dialog box, allowing users to select various printing options such as printer selection, page range, and number of copies.
In this article, we will explore how to implement a print button using JavaScript, examine its functionality, and discuss best practices for enhancing user experience. Whether you’re a beginner or an experienced developer, this guide will help you understand how to effectively use the JavaScript print feature on your web pages.
Understanding window.print()
The window.print()
method is a built-in JavaScript function that triggers the browser’s print dialog. This dialog allows users to print the content currently displayed in the browser window. By using this method, developers can create a print-friendly version of their web pages, ensuring that users can easily print the information they need.
When you call window.print()
, the browser captures the current state of the page and presents it in a print dialog. Users can then choose their preferred printer and set various options, such as orientation and page size. This functionality is particularly useful for generating reports, invoices, or any content that users may want to have in a physical format.
Creating a Print Button in HTML
To create a print button, you can use a simple HTML button element and attach a JavaScript function to it. Below is an example of how to implement this.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Print Page Example</title>
</head>
<body>
<h1>Your Content Here</h1>
<p>This is the content that will be printed.</p>
<button onclick="printPage()">Print this page</button>
<script>
function printPage() {
window.print();
}
</script>
</body>
</html>
In this example, we create a button labeled “Print this page.” When clicked, it calls the printPage()
function, which in turn executes the window.print()
method. This straightforward implementation allows users to print the content of the page with ease.
Customizing Print Styles with CSS
While the window.print()
method is effective, the printed output may not always look as desired. To enhance the print layout, you can use CSS to create print-specific styles. This is achieved by defining styles within a @media print
block in your CSS.
@media print {
body {
font-size: 12pt;
color: black;
}
button {
display: none;
}
}
In this CSS snippet, we specify styles that apply only when printing. The font size is adjusted to ensure readability, and the print button is hidden from the printed output. This customization helps create a cleaner, more professional-looking printout.
Best Practices for Using window.print()
When implementing the window.print()
function, consider the following best practices to enhance user experience:
- Test Across Browsers: Different browsers may render print styles differently. Always test your print functionality in multiple browsers to ensure consistency.
- Provide Clear Instructions: If your page has specific print requirements, provide users with instructions on how to use the print feature effectively.
- Optimize Content for Printing: Ensure that the content you want to print is well-structured and free of unnecessary elements, such as navigation bars or ads.
- Use Print-Specific Styles: As mentioned earlier, utilize CSS to create print-specific styles that enhance the readability and layout of the printed content.
By following these best practices, you can ensure that your users have a seamless experience when printing content from your web pages.
Conclusion
The JavaScript window.print()
method is a powerful tool for enabling printing functionality on your web pages. By implementing a simple print button and customizing print styles with CSS, you can significantly improve the user experience. Remember to test your implementation across different browsers and optimize your content for printing. With these tips and techniques, you can confidently provide users with a reliable and efficient way to print web content.
FAQ
-
What is the purpose of the window.print() method?
The window.print() method triggers the browser’s print dialog, allowing users to print the content of the current web page. -
Can I customize the print layout?
Yes, you can use CSS to create print-specific styles that enhance the layout and readability of the printed content. -
Is the print functionality supported in all browsers?
Most modern browsers support the window.print() method, but it’s always good to test your implementation across different browsers for consistency. -
How can I hide certain elements when printing?
You can use CSS with a @media print query to hide elements, such as buttons or navigation bars, that you do not want to appear in the printed output. -
Can I trigger the print dialog automatically when the page loads?
While it is technically possible, it is generally not recommended due to potential user annoyance. It’s best to provide a clear print button for users to initiate printing.