The preg_match Function in JavaScript
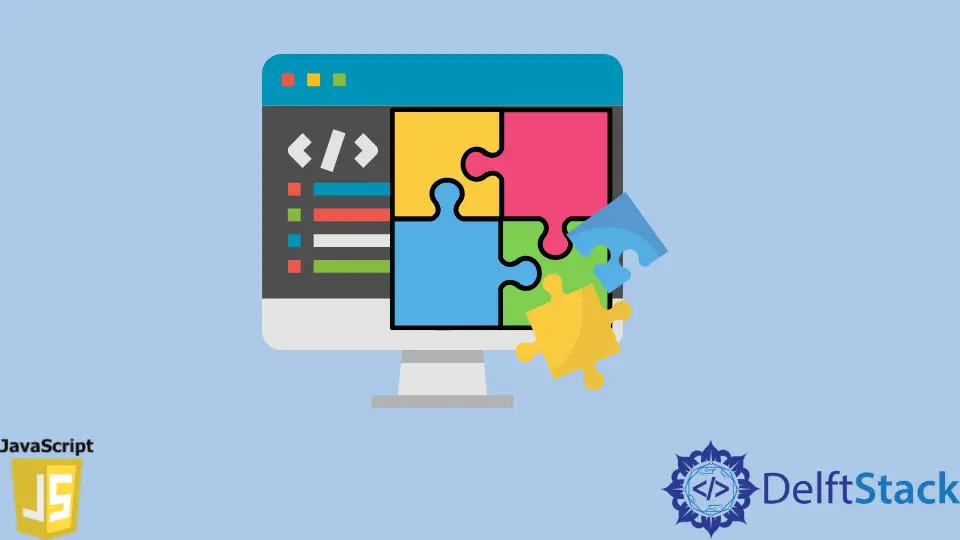
This article will teach the purpose of the preg_match
function in PHP and the possible way to implement the same functionality in JavaScript with an example.
the preg_match
Function in PHP
The preg_match()
is a default function of PHP used to match the strings. This function receives a string and regular expression pattern as arguments and checks if the string portion is matched with a pattern or not; the function will return 0 in the failed case and 1 in the success case.
Basic syntax in PHP:
<?php
$string = "hello world";
$regex_pattern = "/hello/i";
if(preg_match($regex_pattern, $string) == 1) // check the string with regex
{
echo "String matched";
}else{
echo "String not matched";
}
?>
Output:
String matched
Use preg_match
in JavaScript
In JavaScript, we don’t have a built-in function as the preg_match()
function of PHP, but we can achieve the same functionality to check the match portion of a string using a regular expression pattern and JavaScript default method match()
, which is used on string values.
match()
method is used to match a string against a regular expression and returns an array with the matches. It will return null
if no match is found.
Example using JavaScript:
let string = 'hello world';
let regex_pattern = /hello/gi;
let result = string.match(regex_pattern) // check the string with regex
if (result == null) {
console.log('String not matched')
}
else {
console.log('String matched')
}
Output:
String matched
We have declared the 'hello world'
string and a regular expression pattern in the above JavaScript source. Then, we used the match()
method on the hello world
string and passed regular expression.
Now use a conditional statement if the match
method returns null
to say the string is not matched with the regular expression pattern, or else it’s matched.