The Effect of JavaScript Plus Equal
-
JavaScript Plus Equal (
+=
) Between Numbers -
JavaScript Plus Equal (
+=
) Between Strings -
JavaScript Plus Equal (
+=
) Between a Number and a String
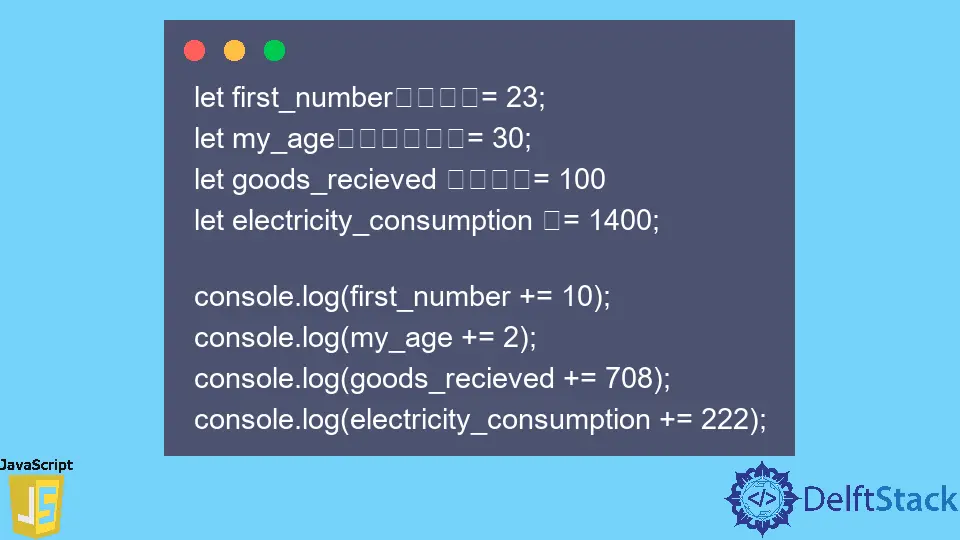
This tutorial will teach you the effect of JavaScript plus equal in the following cases.
- JavaScript plus equal between numbers
- JavaScript plus equal between strings
- JavaScript plus equal between a number and a string
JavaScript Plus Equal (+=
) Between Numbers
When JavaScript plus equal (+=
) sits between two numbers, it’ll add the right number to the left number. However, you should store the left number in a variable; else, you’ll get an error.
In the following example, we’ve created a set of variables whose values are numbers. Afterward, we use JavaScript plus equal between the variables and different numbers.
As a result, JavaScript adds the numbers to our variable values in each situation.
let first_number = 23;
let my_age = 30;
let goods_recieved = 100
let electricity_consumption = 1400;
console.log(first_number += 10);
console.log(my_age += 2);
console.log(goods_recieved += 708);
console.log(electricity_consumption += 222);
Output:
33
32
808
1622
If you have a number array, you can get the sum of the numbers using forEach
and JavaScript plus equal. For example, we have a number array in our next code, and we have declared a variable called total_sum
.
So, when forEach
iterates over the array, JavaScript plus equal adds a number to total_sum
. As a result, we get the sum of the numbers in the array at the end of the iteration.
Meanwhile, the variable total_sum
is an accumulator in this context. We have an array of numbers in the following and use JavaScript plus equal to get their sum.
let my_array = [1, 4, 5, 8, 4, 3, 2, 67, 44];
let total_sum = 0;
my_array.forEach(function(value) {
total_sum += value;
// console.log(total_sum);
});
console.log(total_sum);
Output:
138
JavaScript Plus Equal (+=
) Between Strings
A JavaScript plus equal (+=
) between strings will concatenate the strings. In the following example, we have two strings in different variables.
The first variable has a value of Delft
, and the second variable holds a value of Stack
. So, the concatenation of these strings produces DelftStack
.
let website_first_name = 'Delft';
let website_last_name = 'Stack';
console.log(website_first_name += website_last_name);
Output:
DelftStack
JavaScript Plus Equal (+=
) Between a Number and a String
Using the JavaScript plus equal (+=
) between a number and string will coerce the number into a string. That’s because the +
sign will convert the number into a string in the context of numbers and strings.
Therefore, the code typeof(2 + 'Delft Stack')
will return a string
because the +
sign converted the number 2
into a string.
In the following code, we have a variable called my_number
with a value of 197
. Meanwhile, before adding it to a string, we used the typeof
operator, indicating that my_number
is a number.
However, when we added the string Hello World
to 197
, it was no longer a number
type; instead, it’s now a string
type. So, a subsequent check with the typeof
operator shows that my_number
is now a string.
let my_number = 197;
console.log(
`Before Plus Equal With a String: ${my_number} is a `, typeof (my_number));
my_number += ' Hello World';
console.log(
`After Plus Equal With a String: ${my_number} is a`, typeof (my_number));
// From this point on, my_number is a string
console.log(my_number += 300);
Output:
Before Plus Equal With a String: 197 is a number
After Plus Equal With a String: 197 Hello World is a string
197 Hello World300
Habdul Hazeez is a technical writer with amazing research skills. He can connect the dots, and make sense of data that are scattered across different media.
LinkedIn