How to Ping Server in JavaScript
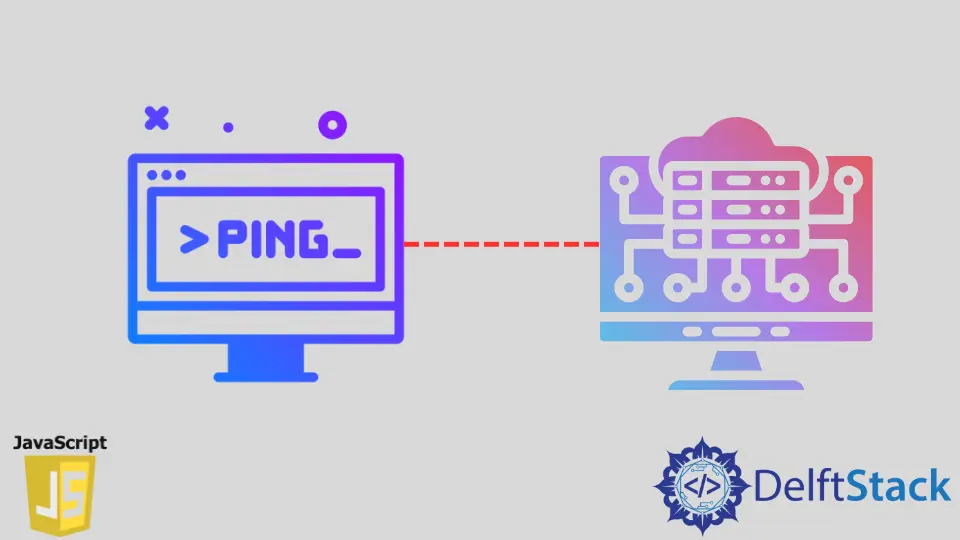
For pinging an URL, we will receive a message from the server if that site is valid or active. If the server verdicts that the URL is on air, it will satisfy a conditional statement.
The basic drive includes an ajax
code boundary that will define the configuration for the request. Let’s direct examine an example to get a grip on the concept.
Use Ajax
to Ping an URL in JavaScript
We will take an input element to enter the user preferred URL, and right after the submit, the onclick
attribute will invoke the ping()
function. We will set the configuration for URL requests in the JavaScript code lines.
We will not have any external if-else
statement to infer the response. Rather we will have multiple statusCode
to return statements based on the servers’ responses.
Code Snippet - index.html
:
<!DOCTYPE html>
<html lang="en">
<head>
<script src="index.js"></script>
<script type="text/javascript" src=
"https://code.jquery.com/jquery-1.7.1.min.js">
</script>
<title>Ping using JavaScript</title>
</head>
<body>
<label for="url">
URL you want to ping:
</label><br>
<input type="text" id="url"
name="url" style="margin: 10px;"><br>
<input type="submit" value="Submit"
onclick="ping()">
</body>
</html>
Code Snippet - index.js
:
function ping() {
// The custom URL
var URL = $('#url').val();
var settings = {
cache: false,
dataType: 'jsonp',
async: true,
crossDomain: true,
url: URL,
method: 'GET',
// For response
statusCode: {
200: function(response) {
console.log('Status 200: Page is up!');
},
400: function(response) {
console.log('Status 400: Page is down.');
},
0: function(response) {
console.log('Status 0: Page is down.');
},
},
};
// Sends the request and observes the response
$.ajax(settings).done(function(response) {
console.log(response);
});
}
Output:
The console responds to the statement with the status 200 and says the page is active, and this certain response is titled pong. This is the way you ping a specific URL to check its validity.