JavaScript parseDouble
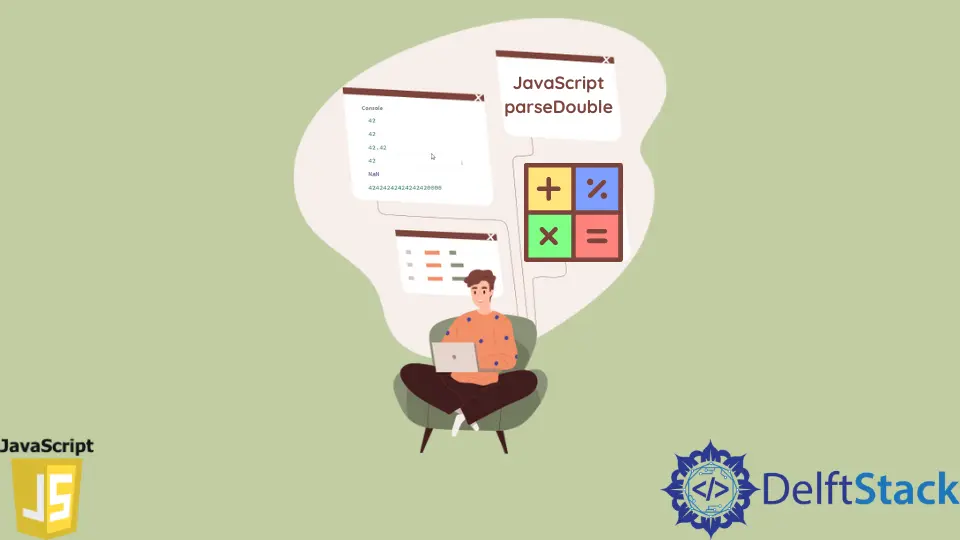
JavaScript only has the number
type of data. Like any other programming language with int
, float
, double
JavaScript, do not follow the rail.
The number type data only works until 16 significant digits. Any digit more than 16 digits will be altered with 0
.
Here, we will see one example that will cover the parsing of an integer, string, and seemingly large number. The instance will depict how parseFloat()
performs the conversion task.
Use parseFloat()
to Parse Other Data Types in JavaScript
Generally, an integer will be parsed to float. But if we deal with a string, there will be some difference parsing the object.
Usually, the parseFloat()
method checks for the first element in a string. If it faces a number, it will parse it and terminate the process.
If a string doesn’t have any number, it will preview NaN
. Let’s check the example for proper visualization of how parseFloat()
works.
Code Snippet:
console.log(parseFloat(42));
console.log(parseFloat('42'));
console.log(parseFloat('42.42'));
console.log(parseFloat('42 7'));
console.log(parseFloat('I\'m not 42 yet'));
console.log(parseFloat(42424242424242424242));
Output:
According to the instance, we have 6 cases to validate the operation of the parseFloat()
method.
The first is an integer, and the expected output will be the exact number. We have two strings in the second and third cases, but both are parsed to number.
The fourth case has two numbers in the string format. As we know, parseFloat()
only encounters the first element, and if it gets a number-like entry, it will be 0.
That’s why it will not print 7
as an answer. So, the next case will show NaN
as the string’s starting doesn’t have any number.
Finally, there is a 20 digit number for parsing, and it will only work with the 16 digits, and the rest are altered with 0
.