How to Parse HTML in JavaScript
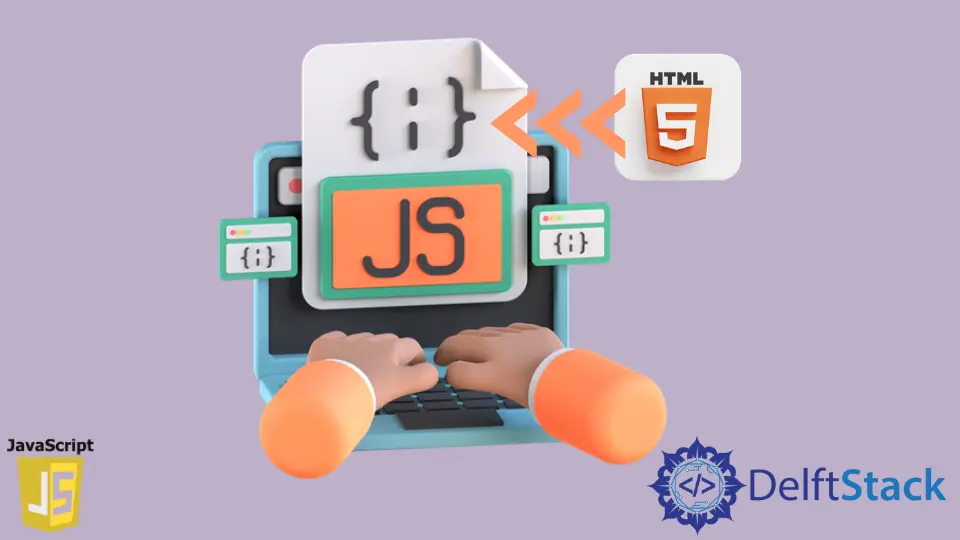
HTML or HyperText Markup Language enables web users to create and structure sections, paragraphs, and links with elements, labels, and attributes.
This post will teach how to parse the HTML content using JavaScript.
Use innerHTML
to Parse HTML in JavaScript
In an HTML document, the document.createElement()
method creates the HTML element specified by tagName
or an HTMLUnknownElement
if tagName
is not recognized.
Syntax:
let element = document.createElement(tagName[, options]);
The tagName
is the string specifying the type of item to create. The node name of the created element is initialized with the value of tagName
.
Do not use qualified names (e.g. html:a
) with this method. In an HTML document, createElement()
converts tagName
to lowercase before creating the element.
For example, an HTML string is passed to your server, and you want to render the content. We have to create a dummy DOM and pass the HTML string to the inner HTML of the freshly created DOM element.
This will give you live nodes of the DOM, and you can access them using any of the methods like getElementsByTagName
, getElementsById
, etc.
var el = document.createElement('html');
el.innerHTML =
'<html><head><title>titleTest</title></head><body><p>Hello World!</p></body></html>';
console.log(el.getElementsByTagName('p').length)
Output:
1
Use DOMParser
to Parse HTML in JavaScript
The DOMParser
interface can analyze the XML or HTML source code in a DOM document. You can do the reverse process and convert a DOM tree to the XMLSerializer
interface in the XML or HTML source.
In the case of an HTML document, you can also replace DOM parts with new DOM trees created by HTML by setting the value of the Element.innerHTML
and outerHTML
properties.
For example, an HTML string is passed to your server, and you want to render the content. We have to first create the DOMParser
object using the constructor.
After creating the object, call the parseFromString
method and input and output document type (creating a dummy DOM).
This will give you live nodes of the DOM, and you can access them using any of the methods like getElementsByTagName
, getElementsById
, etc.
Example:
var parser = new DOMParser();
var htmlDoc = parser.parseFromString(
'<html><head><title>titleTest</title></head><body><p>Hello World!</p></body></html>',
'text/html');
console.log(htmlDoc.getElementsByTagName('p').length)
Output:
1
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn