How to Open File Dialog in JavaScript
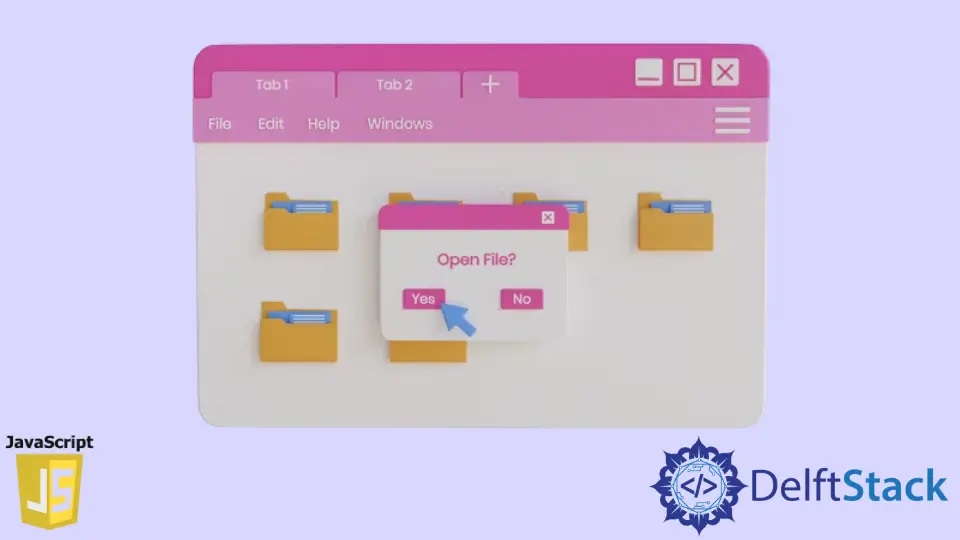
Ever since the type="file"
attribute was introduced in JavaScript, the task of opening a file dialog became effortless. We will discuss the type="file"
attribute in HTML in this article.
Use the type="file"
Attribute in HTML and onchange
Method in JavaScript to Open File Dialog
We will create a button
element in the following instance, and an onclick
attribute will follow this. This will trigger a function in the JavaScript file.
The JavaScript file will have the corresponding function to create an input
element with a type=file
. Specifically, this is the segment where we will open the file dialog to enter the preferable file.
Code Snippet - HTML
:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
</head>
<body>
<button onclick="importD()">Data</button>
<script src="okay.js"></script>
</body>
</html>
Code Snippet - JavaScript
:
function importD() {
let input = document.createElement('input');
input.type = 'file';
input.onchange = _this => {
let files = Array.from(input.files);
console.log(files);
};
input.click();
}
Output:
As seen above, whenever the Data
button will receive a click, it will open up the file dialog, and any file uploaded is previewed in the console lines like in the image below.