How to Accept Only Numbers in Input in JavaScript
-
Use Field Attribute
number
and Set Condition forkeyCode
in JavaScript -
Use Field Attribute
input
and Set Condition forkeyCode
in JavaScript
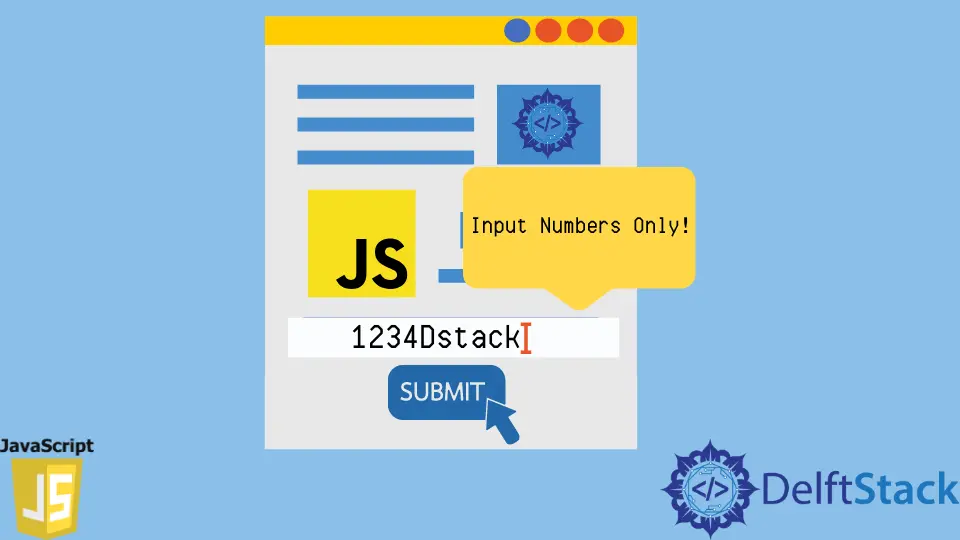
No specific method or property in JavaScript will directly enable the HTML input
field to take only number
type values. Rather there is an attribute number
for the input
field that only takes number type values in the input box.
Another way to perform the task of filtering only the numbers is to set the input
fields type to input
, and this means enabling almost all sorts of possible inputs. This takes into count the alphanumeric values.
The specific conditions implied in the JavaScript code then will filter to accept only the numbers.
We will work with the keypress
event and grab its keyCode
in the following cases. And later, based on our motive, we will set some conditions to validate only the number type data.
One example will have the number
attribute, and the other will have the input
attribute for the input field.
Use Field Attribute number
and Set Condition for keyCode
in JavaScript
Our example will have an input field corresponding to a JavaScript codebase. Initially, we will grab the keyCode
via event.keyCode
or event.which
.
This keyCode
and which
works similarly varies from browser to browser. So, ultimately based on the codes, we will filter the values.
Let’s check the code fence.
<script src="https://code.jquery.com/jquery-3.1.0.js"></script>
<input name="someid" type="number" onkeypress="isNumberKey(event)"/>
<div>
</div>
<script>
function isNumberKey(evt){
var charCode = (evt.which) ? evt.which : evt.keyCode
if (charCode > 31 && (charCode < 48 || charCode > 57))
return false;
return true;
}
$(document).ready(function(){
$("input").keydown(function(event){
$("div").html("Key: " + event.which);
});
});
</script>
Output:
As it can be seen, we have pressed a=65
, b=66
, and c=67
, but those values were not accepted. And later, the values 4
and 2
were placed in the input box.
Use Field Attribute input
and Set Condition for keyCode
in JavaScript
We will similarly set the JavaScript code for our input field in this segment, but this attribute will be set to input
. By default, the Number
type attribute takes numbers and excludes other characters, but in the case of the input
type, we will have the privilege to type other characters like backspace, letters, etc.
Here, our JavaScript code will validate for taking count of the numbers only.
Code Snippet:
<script src="https://code.jquery.com/jquery-3.1.0.js"></script>
<input type="input" id="edit1" size="11" maxlength="10" />
<div>
</div>
<script>
$(document).ready(function(){
$('[id^=edit]').keypress(validateNumber);
});
function validateNumber(event) {
var key = window.event ? event.keyCode : event.which;
if (event.keyCode === 8 || event.keyCode === 46) {
return true;
} else if ( key < 48 || key > 57 ) {
return false;
} else {
return true;
}
};
$(document).ready(function(){
$("input").keydown(function(event){
$("div").html("Key: " + event.which);
});
});
</script>
Output: