How to Create A Noop Function in JavaScript
-
Different Ways to Create
Noop
Function in JavaScript -
Use
Function.prototype
to CreateNoop
Function in JavaScript -
Use Empty Anonymous Function to Create
Noop
Function in JavaScript -
Use Empty Arrow Function to Create
Noop
Function in JavaScript -
Use
_.noop()
to CreateNoop
Function in JavaScript -
Use
$.noop()
to CreateNoop
Function in JavaScript
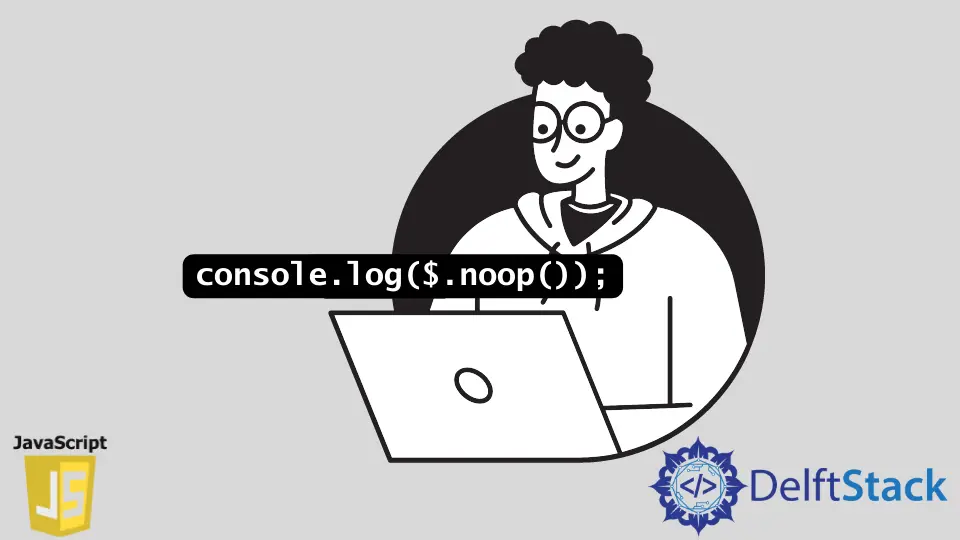
This tutorial highlights the various ways to create a noop
function in JavaScript. The noop
(also known as no operation
) function does nothing; it is very beneficial for the authors who write plugins and perform optional callbacks.
Different Ways to Create Noop
Function in JavaScript
To create a noop
function, we can use Function.Prototype
, _.noop()
, $.noop()
, empty anonymous function, and empty arrow function to get advantage of noop
function.
Let’s explore them one by one by generating random Boolean values. Print it on the console if it is true
; otherwise, it does no operation.
Use Function.prototype
to Create Noop
Function in JavaScript
Example Code:
var ranBoolVal = Math.random() < 0.5;
if (ranBoolVal)
console.log(ranBoolVal)
else Function.prototype;
Here, Function.prototype
is serving as a noop
function. The Function.prototype
is also a function that can be checked using typeof
.
Use Empty Anonymous Function to Create Noop
Function in JavaScript
Example Code:
var ranBoolVal = Math.random() < 0.5;
if (ranBoolVal) {
console.log(ranBoolVal)
} else {
const noop = function() {};
}
Here, the empty anonymous function is acting like a noop
function. We can also replace the statement const noop = function () {};
of else
section with function noop() {}
to get the same effect.
Use Empty Arrow Function to Create Noop
Function in JavaScript
Example Code:
var ranBoolVal = Math.random() < 0.5;
if (ranBoolVal) {
console.log(ranBoolVal)
} else {
const noop = () => {};
}
Use _.noop()
to Create Noop
Function in JavaScript
Example Code:
var ranBoolVal = Math.random() < 0.5;
if (ranBoolVal)
console.log(ranBoolVal)
else console.log(_.noop());
We are using a _.noop()
from the Underscore.js
library. The _.noop()
returns undefined
, which does nothing irrespective of the passing parameters.
See the following example.
var ranBoolVal = Math.random() < 0.5;
if (ranBoolVal)
console.log(ranBoolVal)
else console.log(_.noop(3 + 4));
Use $.noop()
to Create Noop
Function in JavaScript
Example Code:
var ranBoolVal = Math.random() < 0.5;
if (ranBoolVal)
console.log(ranBoolVal)
else console.log($.noop());
We use jQuery’s function noop()
, which takes no arguments and returns undefined
.