JavaScript Negative Number
- Understanding Negative Numbers in JavaScript
- Method 1: Direct Assignment of Negative Numbers
- Method 2: Mathematical Operations to Generate Negative Numbers
- Method 3: Using the Math Library to Create Negative Values
- Conclusion
- FAQ
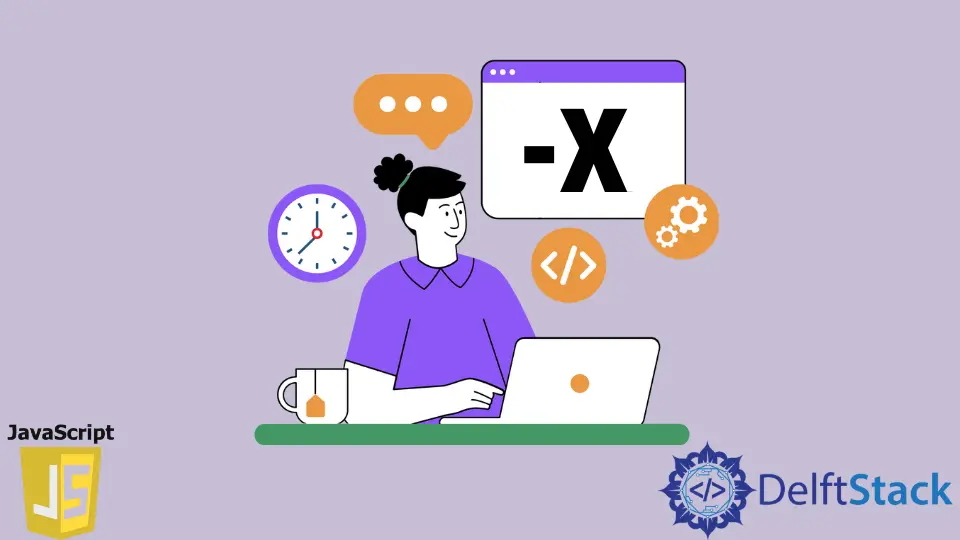
In today’s article, we will learn how to get a negative number in JavaScript. Understanding how to work with negative numbers is crucial for any developer, especially when dealing with mathematical operations, data validation, or even game development. JavaScript, being a versatile and widely-used programming language, provides various ways to handle numbers, including negative values. Whether you are manipulating user input or performing calculations, knowing how to effectively manage negative numbers can enhance your programming skills significantly. So, let’s dive into the world of JavaScript and discover how to create and manage negative numbers seamlessly.
Understanding Negative Numbers in JavaScript
Before we delve into the methods to create negative numbers in JavaScript, it’s essential to understand what a negative number is. A negative number is any number less than zero, typically represented with a minus sign (-). In JavaScript, negative numbers can be used in various contexts, such as arithmetic operations, comparisons, and data storage.
JavaScript treats numbers as floating-point values, which means both integers and decimals can be negative. For instance, -5, -3.14, and -1000 are all valid negative numbers in JavaScript. This flexibility allows developers to implement complex logic and calculations without worrying about the data type.
Method 1: Direct Assignment of Negative Numbers
The simplest way to create a negative number in JavaScript is through direct assignment. You can assign a negative value directly to a variable using the minus sign. This method is straightforward and works well for both integers and floating-point numbers.
let negativeInteger = -10;
let negativeFloat = -3.14;
console.log(negativeInteger);
console.log(negativeFloat);
Output:
-10
-3.14
In this example, we created two variables: negativeInteger
and negativeFloat
. The first variable holds a negative integer value of -10, while the second holds a negative floating-point value of -3.14. When we log these variables to the console, we see the expected negative numbers. This method is simple yet effective, making it the go-to choice for many developers when they need to work with negative numbers.
Method 2: Mathematical Operations to Generate Negative Numbers
Another way to obtain negative numbers in JavaScript is through mathematical operations. You can perform arithmetic operations that result in a negative value. This method is particularly useful when dealing with calculations that may yield negative results.
let result = 5 - 10;
let anotherResult = -1 * 3;
console.log(result);
console.log(anotherResult);
Output:
-5
-3
In this code snippet, we first subtract 10 from 5, which gives us -5. Next, we multiply -1 by 3, resulting in -3. Both operations demonstrate how you can derive negative numbers through basic arithmetic. This method is particularly handy when you need negative values as part of a calculation or algorithm, allowing for dynamic generation of negative numbers based on user input or other variables.
Method 3: Using the Math Library to Create Negative Values
JavaScript’s built-in Math
library offers various mathematical functions that can also be used to create negative numbers. Functions like Math.abs()
can be paired with multiplication to achieve negative results. This method is particularly useful for more complex scenarios where negative numbers are generated from various calculations.
let positiveValue = Math.abs(-25);
let negativeValue = -Math.abs(25);
console.log(positiveValue);
console.log(negativeValue);
Output:
25
-25
In this example, we first use Math.abs()
to get the absolute value of -25, which is 25. Then, we negate that absolute value, resulting in -25. This method showcases the versatility of the Math
library in JavaScript. By leveraging built-in functions, developers can create robust applications that require dynamic generation of negative numbers based on various conditions or calculations.
Conclusion
In conclusion, working with negative numbers in JavaScript is simple and intuitive. By using direct assignment, mathematical operations, or the Math library, developers can easily create and manipulate negative values. Understanding how to handle negative numbers is essential for any programmer, as it opens up various possibilities for calculations, data validation, and more. As you continue your journey in JavaScript, keep these methods in mind to enhance your coding skills and create more dynamic applications.
FAQ
-
How do I check if a number is negative in JavaScript?
You can check if a number is negative by using a simple conditional statement likeif (number < 0)
. -
Can I convert a positive number to a negative number in JavaScript?
Yes, you can convert a positive number to a negative number by multiplying it by -1, likelet negativeNumber = positiveNumber * -1
.
-
What happens if I try to add a negative number to a positive number?
Adding a negative number to a positive number will reduce the value of the positive number. For example,5 + (-3)
equals2
. -
Are negative numbers treated differently in JavaScript?
No, negative numbers are treated as regular numbers in JavaScript. They can be used in arithmetic operations just like positive numbers. -
Can I use negative numbers in arrays?
Yes, you can store negative numbers in arrays just like any other number type in JavaScript.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn