Named Parameters in JavaScript
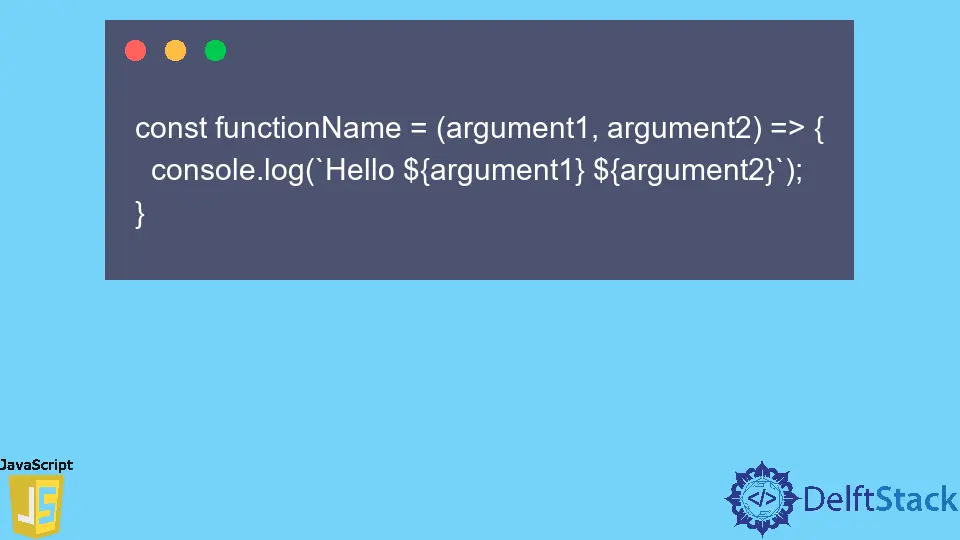
In today’s post, we’ll look at named parameters in JavaScript.
Named Parameters in JavaScript
The main point to take away from positional arguments is that the argument’s name is meaningless. The order in which arguments are passed is the only thing that matters.
const functionName = (argument1, argument2) => {
console.log(`Hello ${argument1} ${argument2}`);
}
Since it is difficult to mix up the order of arguments, it usually works perfectly for situations where you send one or two arguments. However, it would be difficult to remember the sequence of arguments to pass if you had to make a call that requires an n
number of arguments.
By default, named arguments are not supported by JavaScript. However, you may accomplish a similar task by using object literals and destructuring.
Even if you have default values set up, you can prevent errors when calling the function without any parameters by assigning the object to the empty object.
Since the function is no longer dependent on the order in which the arguments are supplied, we may also exclude the arguments we don’t wish to pass by using the pattern of the named argument.
const namedParamFunction = ({argument1, argument2, argument3} = {}) => {
console.log(argument1, argument2, argument3)
} namedParamFunction({argument3: 'DelftStack!', argument1: 'Hello'});
In conclusion, we’d argue that named arguments are a strong pattern that is now widely used, but you don’t necessarily need to use them. You might even combine the two at times.
Run the above code line in any browser compatible with JavaScript. It will display the following outcome:
"Hello DelftStack Users!"
"Hello", undefined, "DelftStack!"
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn