Modulo Operator(%) in JavaScript
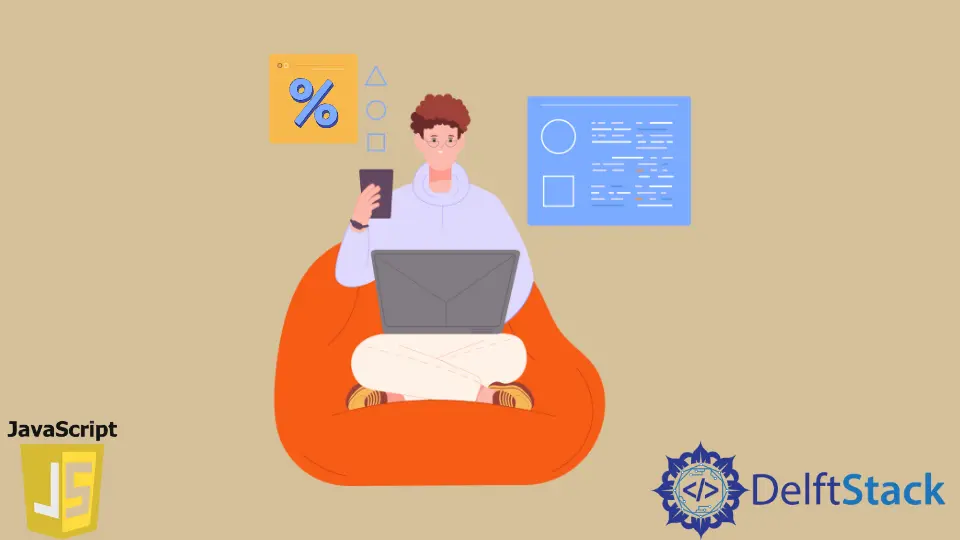
This tutorial teaches how to use the modulo operator %
in JavaScript.
Remainder Operator %
in JavaScript
It gives the remainder left over when one number (dividend)
is divided by another number (divisor)
. This operator is not the same as the modulo
operator in other languages because it serves a different purpose. Their results are the same only for a positive dividend, but if we have a negative dividend a
and a modulo operator is applied over it, then the results will be completely different. The result obtained by the expression ( (a % n) + n) % n
using the remainder operator in JavaScript is the same as the result obtained using the modulo operator in a % n
.
Example of Using Remainder Operator %
in JavaScript
Modulo With Positive Dividend
1 % -2 // 1
2 % 3 // 2
5.5 % 2 // 1.5
12 % 5 // 2
1 % 2 // 1
Remainder With Negative Dividend
-12 % 5 // -2
- 1 % 2 // -1
- 4 % 2 // -0
Remainder With NaN
NaN % 2 // NaN
Remainder With Infinity
Infinity % 2 // NaN
Infinity % 0 // NaN
Infinity % Infinity // NaN
Applications
Is a Number Odd or Even?
We can check if an integer is even by checking if it is divisible by 2
. We can use the modulo operator’s return value. If it is 0
, it means the number is even.
function isEven(n) {
return n % 2 === 0;
}
isEven(6); // true
isEven(3); // false
the Fractional Part of a Number
We can do this simply by calculating n % 1
.
function getFractionalPart(n) {
return n % 1;
}
getFractionalPart(2.5); // 0.5
Convert Minutes to Hours
When given a number n
that represents the number of minutes and we want to convert it to hours and minutes, we can use the modulo operator.
const minutesToHoursAndMinutes = n =>
({hours: Math.floor(n / 60), minutes: n % 60});
minutesToHoursAndMinutes(123); // { hours: 2, minutes: 3 }
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedIn