Module Loader in JavaScript
- What is a Module Loader?
- Using a Module Loader: Example with RequireJS
- Module Loading with ES6
- Conclusion
- FAQ
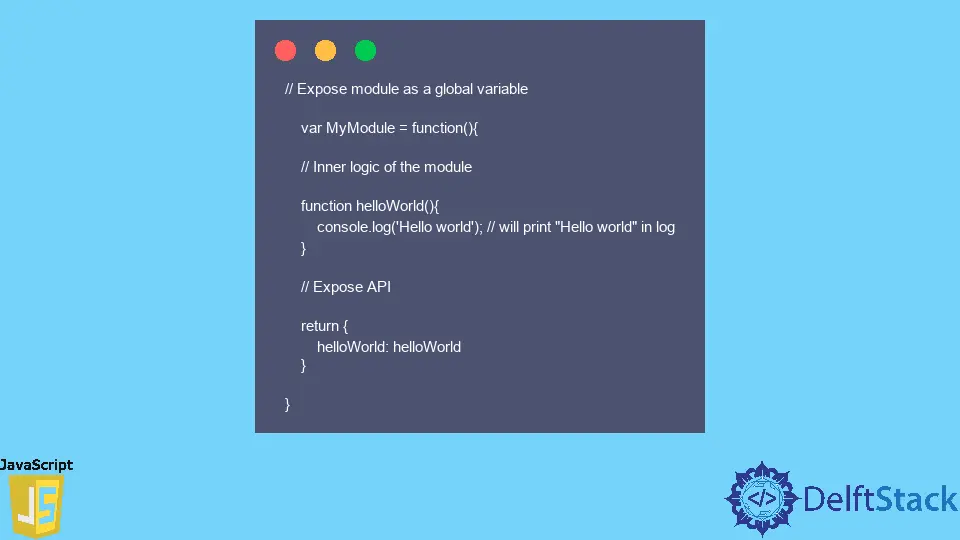
In today’s fast-paced development environment, JavaScript has evolved significantly, leading to the need for better organization and modularity in code. This is where module loaders come into play. A module loader is a tool that helps developers load and manage JavaScript modules efficiently, making it easier to maintain and scale applications.
In this article, we will explore what a module loader is, how it works, and provide an example of using a module loader to load JavaScript modules in the main JavaScript file. Whether you’re a beginner or an experienced developer, understanding module loaders is crucial for writing clean and maintainable code.
What is a Module Loader?
A module loader is a JavaScript library or framework that allows developers to load modules on demand. This means that instead of loading all JavaScript files at once, you can load only the necessary modules when they are needed. This approach enhances performance and reduces the initial load time of web applications.
Module loaders typically follow the CommonJS or AMD (Asynchronous Module Definition) standards, which define how modules should be structured and how they can be loaded. With the introduction of ES6 modules, native support for module loading has also been integrated into modern browsers. However, using a module loader can still be beneficial for compatibility and advanced features.
Using a Module Loader: Example with RequireJS
One of the most popular module loaders is RequireJS, which implements the AMD pattern. Let’s see how to use RequireJS to load JavaScript modules in your main JavaScript file.
First, you need to include the RequireJS library in your HTML file:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>RequireJS Example</title>
<script data-main="app" src="https://cdnjs.cloudflare.com/ajax/libs/require.js/2.3.6/require.min.js"></script>
</head>
<body>
</body>
</html>
Next, create a module named math.js
that exports a simple addition function:
// math.js
define([], function() {
return {
add: function(a, b) {
return a + b;
}
};
});
Now, create the main file app.js
where you will load the math.js
module:
// app.js
require(['math'], function(math) {
var result = math.add(5, 3);
console.log('The result is: ' + result);
});
Output:
The result is: 8
In this example, we first define a module called math.js
using the define
function. This module exports an object containing an add
function. In app.js
, we use the require
function to load the math
module and call the add
function. The result is then logged to the console.
Using RequireJS allows you to keep your code organized and modular, making it easier to manage dependencies and maintain your application.
Module Loading with ES6
With the introduction of ES6, JavaScript now supports native module loading. This allows developers to use the import
and export
keywords to manage modules without the need for a separate loader. Here’s how you can use ES6 modules in your JavaScript files.
First, create a module named math.js
:
// math.js
export function add(a, b) {
return a + b;
}
Next, create the main file app.js
to import the add
function:
// app.js
import { add } from './math.js';
const result = add(5, 3);
console.log('The result is: ' + result);
Output:
The result is: 8
In this example, we use the export
keyword to expose the add
function in math.js
. In app.js
, we import the function using the import
statement. The result is calculated and logged to the console just like in the previous example.
Using ES6 modules is straightforward and integrates seamlessly with modern browsers. It eliminates the need for additional libraries like RequireJS, making your code cleaner and easier to read.
Conclusion
In conclusion, module loaders play a vital role in modern JavaScript development. They allow developers to load and manage modules efficiently, enhancing code organization and performance. Whether you choose to use a module loader like RequireJS or opt for the native ES6 module system, understanding how to work with modules is essential for any JavaScript developer. By adopting modular programming practices, you can create scalable and maintainable applications that are easier to debug and extend.
FAQ
-
What is a module loader in JavaScript?
A module loader is a tool that helps load JavaScript modules on demand, improving code organization and performance. -
What are some popular module loaders?
Some popular module loaders include RequireJS, SystemJS, and Webpack. -
How do I use ES6 modules?
You can use ES6 modules by using theimport
andexport
keywords in your JavaScript files. -
What are the benefits of using a module loader?
Using a module loader helps manage dependencies, improves performance, and enhances code maintainability. -
Can I use module loaders in older browsers?
Yes, some module loaders like RequireJS provide compatibility for older browsers by using polyfills and transpilation.