JavaScript Matrix Multiplication
- Use Logical Drive to Perform Matrix Multiplication in JavaScript
- Use Methods to Calculate Matrix Multiplication in JavaScript
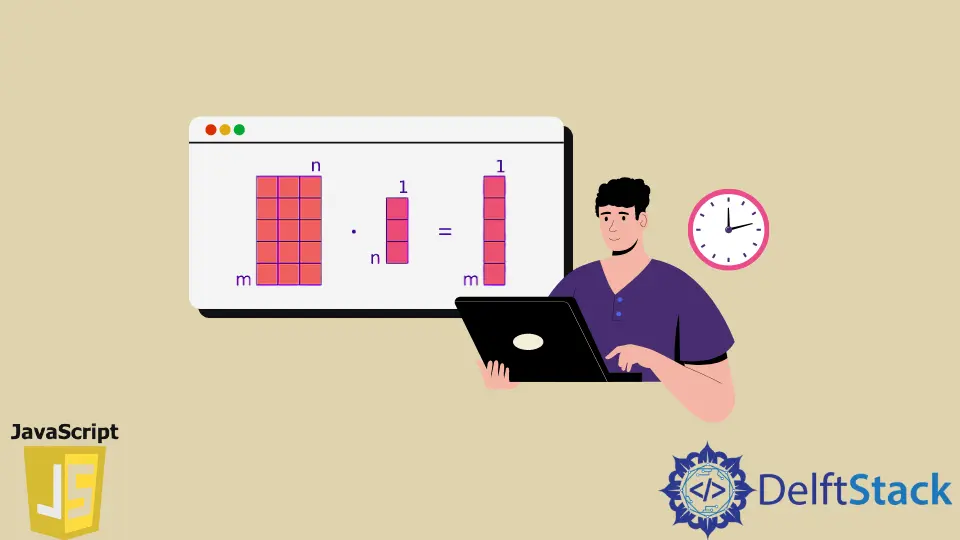
In JavaScript, we not only deal with web-development solutions, but we can also have the flexibility of performing basic calculations. After all, it is a programming language.
We will take a nested array to enable a matrix pattern in JavaScript. This will represent the rows and columns effortlessly.
In matrix multiplication, we need to set some predefined conditions. Such as, if the matrix A
has row_1 = 2
, column_1 = 3
and matrix B
has row_2 = 3
, column_2 = 2
then the matrix duo are compatible to be multiplied.
The basic condition column_1
has to be equal to row_2
. And the result will have an order of 2x2
.
We will examine two examples to explain the task.
Use Logical Drive to Perform Matrix Multiplication in JavaScript
In this instance, we will generate a nested loop to traverse the whole array, aka the matrix array. We will check the row
elements of the first matrix and calculate the basic sum and multiplication operation with the second matrix’s column
.
Let’s check the code fence and try to understand.
function multiplyMatrices(m1, m2) {
var result = [];
for (var i = 0; i < m1.length; i++) {
result[i] = [];
for (var j = 0; j < m2[0].length; j++) {
var sum = 0;
for (var k = 0; k < m1[0].length; k++) {
sum += m1[i][k] * m2[k][j];
}
result[i][j] = sum;
}
}
return result;
}
let m1 = [[1, 2, 42], [4, 5, 7]];
let m2 = [[1, 2], [3, 4], [42, 7]];
var result = multiplyMatrices(m1, m2)
console.table(result)
Output:
Use Methods to Calculate Matrix Multiplication in JavaScript
We will use the map()
and reduce()
methods to draw the result. The iteration process does not need to be explicitly defined, but the map()
performs by default.
The following codebase explains the rest of the explanation.
let MatrixProd = (A, B) => A.map(
(row, i) => B[0].map(
(_, j) => row.reduce((acc, _, n) => acc + A[i][n] * B[n][j], 0)))
let A = [[1, 2, 3], [4, 5, 6]];
let B = [[1, 2], [3, 4], [5, 6]];
console.table(MatrixProd(A, B));
Output: