How to Load Image From URL in JavaScript
- Using the Image Constructor
- Using the Fetch API
- Dynamic Image Loading with Event Listeners
- Conclusion
- FAQ
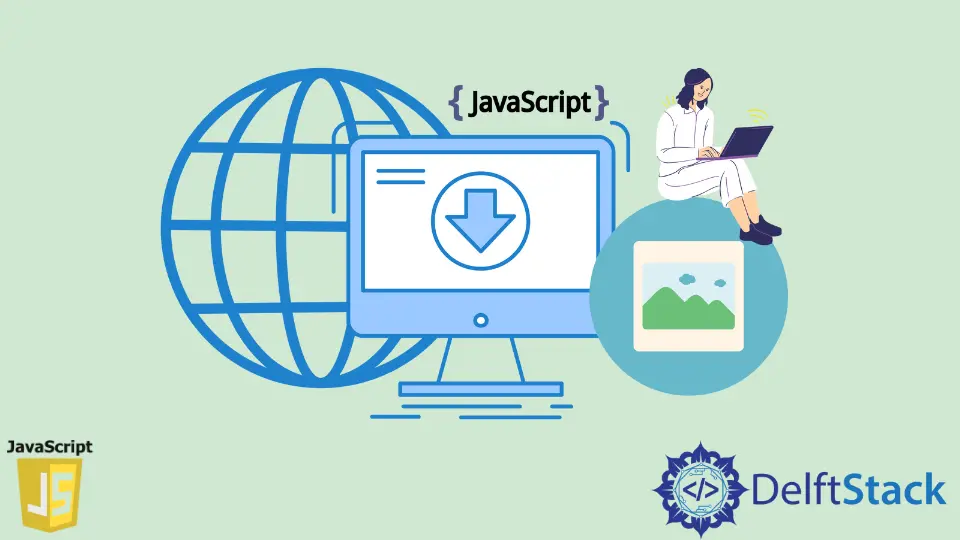
In today’s post, we’ll learn about loading images from specified URLs in JavaScript. Whether you’re building a web application, a simple webpage, or just experimenting with JavaScript, knowing how to load images dynamically can enhance your project significantly. Loading images from URLs can be particularly useful when you want to display user-generated content, pull images from an API, or simply manage images more effectively.
In this article, we will explore different methods to load images from URLs using JavaScript, providing clear examples and explanations to help you understand the process. Let’s dive in!
Using the Image Constructor
One of the simplest ways to load an image from a URL in JavaScript is by using the Image
constructor. This method allows you to create a new image object and set its source to a URL. It’s straightforward and efficient for loading images dynamically.
const imageUrl = "https://example.com/image.jpg";
const img = new Image();
img.src = imageUrl;
img.onload = function() {
document.body.appendChild(img);
};
img.onerror = function() {
console.error("Failed to load image from URL");
};
When you create a new Image
object, you can set its src
property to the desired URL. The onload
event is triggered once the image is successfully loaded, allowing you to append it to the document or perform other actions. Conversely, if there’s an issue loading the image, the onerror
event will catch the error, enabling you to handle it gracefully.
Using this method is particularly beneficial when you want to preload images or manage them dynamically based on user interactions or API responses. The Image
constructor provides a clean and efficient way to work with images in JavaScript.
Output:
Image loaded successfully and appended to the document body.
Using the Fetch API
Another powerful method for loading images from a URL is by utilizing the Fetch API. This approach allows you to make network requests and handle responses in a more flexible way. Although Fetch is typically used for fetching data, it can also be used to retrieve image blobs.
const imageUrl = "https://example.com/image.jpg";
fetch(imageUrl)
.then(response => {
if (!response.ok) {
throw new Error("Network response was not ok");
}
return response.blob();
})
.then(imageBlob => {
const img = document.createElement("img");
img.src = URL.createObjectURL(imageBlob);
document.body.appendChild(img);
})
.catch(error => {
console.error("There was a problem with the fetch operation:", error);
});
In this example, we first fetch the image from the specified URL. If the response is successful, we convert it into a blob using the blob()
method. Then, we create an image element and set its source to a URL created from the blob using URL.createObjectURL()
. Finally, we append the image to the document body.
Using the Fetch API to load images can be particularly useful when you’re working with images stored on different servers or when you need to handle CORS (Cross-Origin Resource Sharing) issues. It provides flexibility and control over the image-loading process.
Dynamic Image Loading with Event Listeners
Sometimes, you may want to load images based on specific user actions, such as clicking a button. By using event listeners, you can dynamically load images from URLs when required.
const button = document.createElement("button");
button.textContent = "Load Image";
document.body.appendChild(button);
button.addEventListener("click", function() {
const imageUrl = "https://example.com/image.jpg";
const img = new Image();
img.src = imageUrl;
img.onload = function() {
document.body.appendChild(img);
};
img.onerror = function() {
console.error("Failed to load image from URL");
};
});
In this code, we create a button that, when clicked, will load an image from a specified URL. The Image
constructor is used again to create the image object, and we handle the loading process with onload
and onerror
events. This method is particularly effective for interactive applications where images are loaded based on user input.
Using event listeners not only enhances user experience but also optimizes resource loading, as images are only fetched when necessary. This approach can significantly improve performance in applications with multiple images.
Conclusion
Loading images from URLs in JavaScript is a fundamental skill that can greatly enhance your web projects. Whether you choose to use the Image
constructor, the Fetch API, or event listeners, each method provides unique advantages depending on your specific needs. By mastering these techniques, you can create more dynamic and engaging web applications. Remember to handle errors gracefully to improve user experience, and don’t hesitate to experiment with different methods to find what works best for your project.
FAQ
- How do I load an image from a URL in JavaScript?
You can load an image by using the Image constructor, the Fetch API, or by setting the src attribute of an img element.
-
What should I do if the image fails to load?
You can use the onerror event handler to catch loading errors and display a message or a fallback image. -
Can I load images from different domains?
Yes, you can load images from different domains, but ensure that the server allows cross-origin requests. -
Is there a way to preload images in JavaScript?
Yes, you can preload images using the Image constructor by creating an image object and setting its src property. -
What is the Fetch API?
The Fetch API is a modern way to make network requests in JavaScript, allowing you to retrieve resources like images, JSON, and more.
Shraddha is a JavaScript nerd that utilises it for everything from experimenting to assisting individuals and businesses with day-to-day operations and business growth. She is a writer, chef, and computer programmer. As a senior MEAN/MERN stack developer and project manager with more than 4 years of experience in this sector, she now handles multiple projects. She has been producing technical writing for at least a year and a half. She enjoys coming up with fresh, innovative ideas.
LinkedIn