How to Get the Length of Object in JavaScript
Kirill Ibrahim
Feb 02, 2024
-
Use the
Object.keys()
Method to Get the Length of an Object in JavaScript -
Use
for...
Loop to Get the Length of an Object in JavaScript
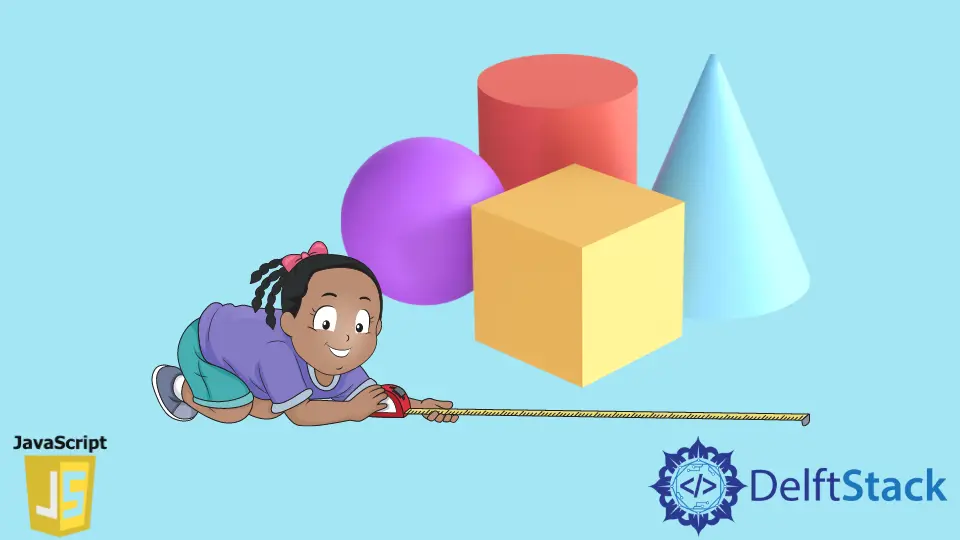
We want to get the length of an object in JavaScript, but the Object
doesn’t have a length
property. Only arrays and strings have the length
property.
let object1 = {name: 'Mark', age: 30};
let string1 = 'Delftstack';
let array1 = [1, 2, 3];
console.log(object1.length);
console.log(string1.length);
console.log(array1.length);
Output:
undefined
10
3
We will introduce different methods to get the length of an object in JavaScript.
Use the Object.keys()
Method to Get the Length of an Object in JavaScript
The Object.keys()
method returns an array of properties of the Object
. We use the length
property to get the number of keys.
Example:
const getLengthOfObject =
(obj) => {
let lengthOfObject = Object.keys(obj).length;
console.log(lengthOfObject);
}
getLengthOfObject({id: 1, name: 'Mark', age: 30});
Output:
3
Use for...
Loop to Get the Length of an Object in JavaScript
It iterates the properties of the object and will increase the counter of properties in the loop.
Example:
const getLengthOfObject =
(obj) => {
let length0fObject = 0;
for (let key in obj) {
length0fObject++;
}
console.log(length0fObject);
return length0fObject;
}
getLengthOfObject({id: 1, name: 'Mark', age: 30});
Output:
3
Example:
const getLengthOfObject =
(obj) => {
let length0fObject = 0;
for (let key in obj) {
if (obj.hasOwnProperty(key)) {
length0fObject++;
}
}
console.log(length0fObject);
return length0fObject;
}
getLengthOfObject(
{id: 1, name: 'Mark', age: 30, country: 'USA', job: 'software developer'});
The hasOwnProperty()
function is a built-in function in JavaScript is used to check if the object owns the specified property. it returns a true
or false
depending on if the object has the specified property or not.