How to Store Key-Value Array in JavaScript
Kirill Ibrahim
Feb 02, 2024
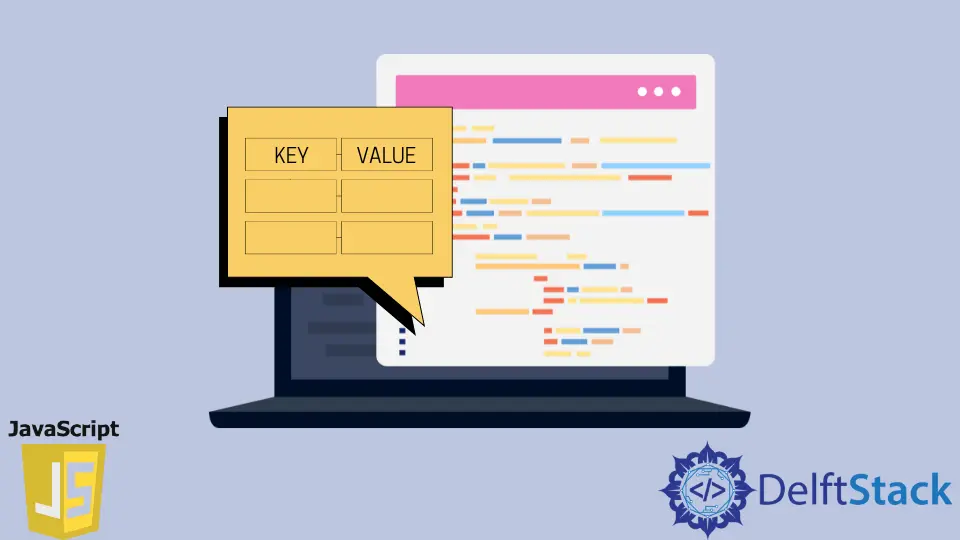
Arrays in JavaScript are single variables storing different elements. We could need them to store a list of elements, and each element has an index to access them by it. JavaScript has different methods to store a key-value array.
Use JavaScript Object
to Store a Key-Value Array
The JavaScript Object
is an entity with properties, and each property has value, so key-value terminology can work on it.
Example:
let obj1 = {id: 1, name: 'Mark', age: 30, country: 'USA'};
obj1.city = 'New York';
obj1['job'] = 'software developer';
console.log(obj1);
Output:
{
age: 30
city: "New York"
country: "USA"
id: 1
job: "software developer"
name: "Mark"
}
We can loop through it:
let obj1 = {id: 1, name: 'Mark', age: 30, country: 'USA'};
obj1.city = 'New York';
obj1['job'] = 'software developer';
for (let key in obj1) {
console.log(key + ' => ' + obj1[key]);
}
Output:
id => 1
name => Mark
age => 30
country => USA
city => New York
job => software developer
If we have an array, we can loop through the array one by one, add the keys from each element’s index, and the corresponding values in the Object.
let arr1 = ['delfstack', 'Computer', 'Science'];
let obj1 = {};
for (let i = 0; i < arr1.length; i++) {
obj1[i] = arr1[i];
}
for (let key of Object.keys(obj1)) {
console.log(key + ' => ' + obj1[key])
}
Output:
0 => delfstack
1 => Computer
2 => Science
Use JavaScript Map to Store a Key-Value Array
Map
is just like an Object
. It is a list of keyed data items. The difference is that Map
allows any type of keys.
Syntax of JavaScript Map
let map = new Map();
- Store key => value:
map.set('name', 'mark');
Example of Java Map
let arr1 = ['delfstack', 'Computer', 'Science'];
let map = new Map();
for (let i = 0; i < arr1.length; i++) {
map.set(i, arr1[i]);
}
for (let key of map.keys()) {
console.log(key + ' => ' + map.get(key))
}
Output:
0 => delfstack
1 => Computer
2 => Science
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript