How to Jump to Anchor in JavaScript
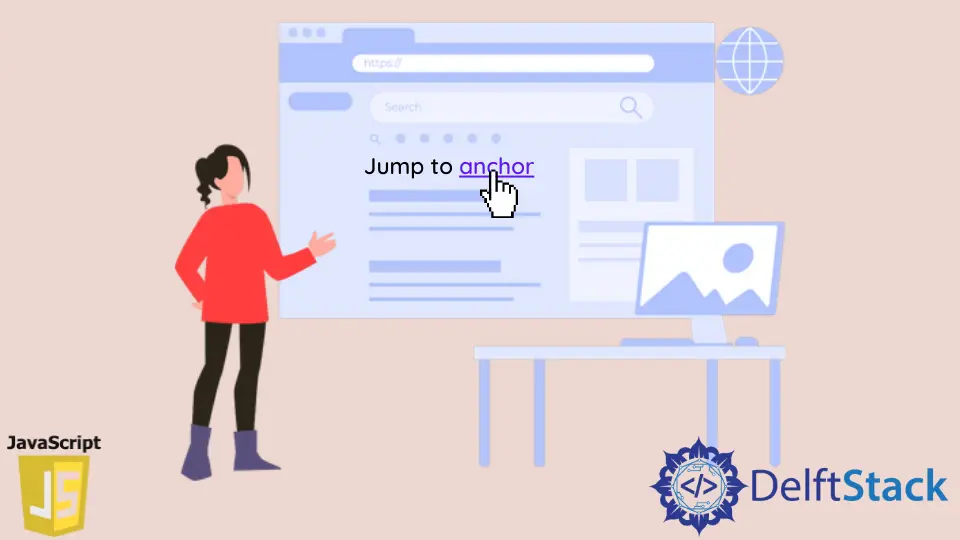
On websites, we usually observe that if we want to navigate to a specific portion or content of a webpage, we need to scroll up and down to redirect and focus on that targeted element.
The basic idea behind anchor jumping is to auto-navigate or scroll to the desired content with a single click.
Jump to Anchor in HTML
In HTML, we define id
to the tags like heading, image, and paragraph, as shown below.
htmlCopy<tag id="anchor-id">Name of Section</tag>
And to redirect to that tagged content in HTML, we use the anchor
tag with hash reference of content id as shown below.
htmlCopy<a href="#anchor-id">navigate to section</a>.
Users can auto-navigate to the desired content by clicking the navigate to section
anchor tag.
Anchor Jumping in JavaScript
In JavaScript, we can declare a single function that can handle all anchors jump of a webpage to navigate specific content or webpage portion.
We will declare a function in which we will receive anchor id
as an argument, and with the help of that id, we will redirect the user to the target element using location.href
.
Here is an example of an anchor jump using JavaScript.
HTML:
htmlCopy<body>
<h2 id="one">One Heading</h2>
<p class="main-content">
Lorem Ipsum is a printing and typesetting industry dummy text. Since the 1500s, when an unknown printer scrambled a galley of type to construct a type specimen book, Lorem Ipsum has been the industry's standard sham text.
</p>
<h2 id="two">Two Heading</h2>
<p class="main-content">
Lorem Ipsum is a printing and typesetting industry dummy text. Since the 1500s, when an unknown printer scrambled a galley of type to construct a type specimen book, Lorem Ipsum has been the industry's standard sham text.
</p>
<a onclick="jumpTo('one');">Navigate to One</a>
<a onclick="jumpTo('two');">Navigate to two</a>
</body>
JavaScript:
htmlCopy<script>
function jumpTo(anchor_id){
var url = location.href; //Saving URL without hash.
location.href = "#"+anchor_id; //Navigate to the target element.
history.replaceState(null,null,url); //method modifies the current history entry.
}
</script>
- The example above shows the HTML source that contains multiple paragraph content with separate headings.
- We have assigned ids to the headings one and two used for navigation.
- We have used multiple anchor tags:
Navigate to One
andNavigate to two
, in which we have called the functionjumpTo()
on click. - Function
jumpTo()
will receive stringid
as an argument. - We have declared the function
jumpTo(anchor_id)
in the JavaScript code to get the anchor id and generate a URL without the hash. - We navigate the target element by assigning
location.href
to the passed anchor id with concatenating hash. history.replaceState
method is used for modifying the current history entry.