Difference Between Inline and Anonymous Functions in JavaScript
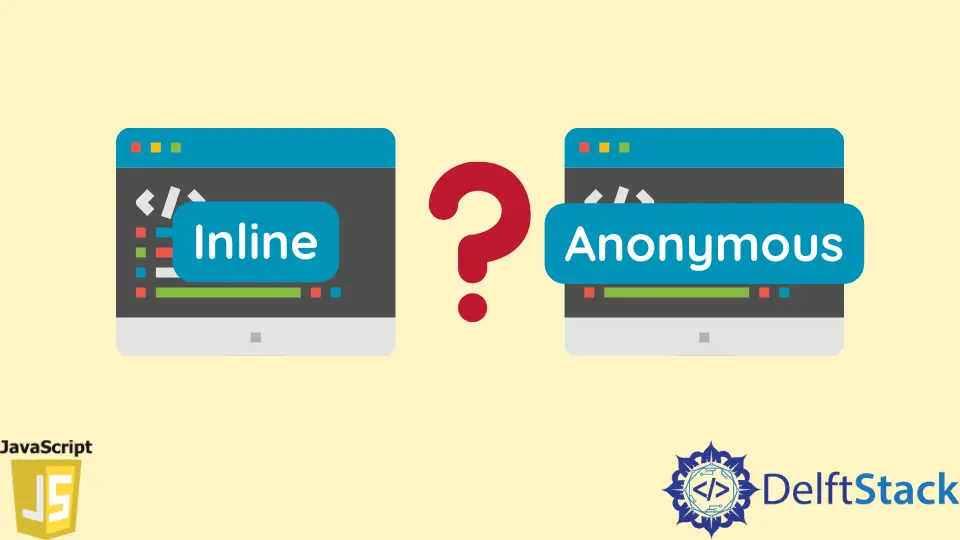
In this article, you will understand the differences between inline and anonymous functions in JavaScript and how to use them in code.
Difference Between Inline and Anonymous Functions in JavaScript
A JavaScript inline function is an anonymous function within a variable. It is always called with the URL of the anonymous function.
They are created at run time and are not significant.
Anonymous and inline functions are nearly identical in that they are both produced at runtime. On the other hand, an inline function is allocated to a variable and thus may be reused.
In this sense, inline functions behave similarly to normal functions.
Inline Function Example:
var samelineFunc = function() {
alert('inline function');
};
$('#inline_func_c').click(samelineFunc);
Anonymous Function Example:
$('#anon_func_b').click(function() {
alert('anonymous function');
});
Output 1:
When you run the code above, you will see something like this:
Here, you can see two buttons: Button_1
is working as an anonymous function, and Button_2
is working as an inline function. You can see in the above code that we can call the anonymous function directly.
Output 2:
On the other hand, you can see a variable created for the inline function so that it can be used anywhere at any time.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn