How to Check if a Variable Is Not Null in JavaScript
Harshit Jindal
Feb 02, 2024
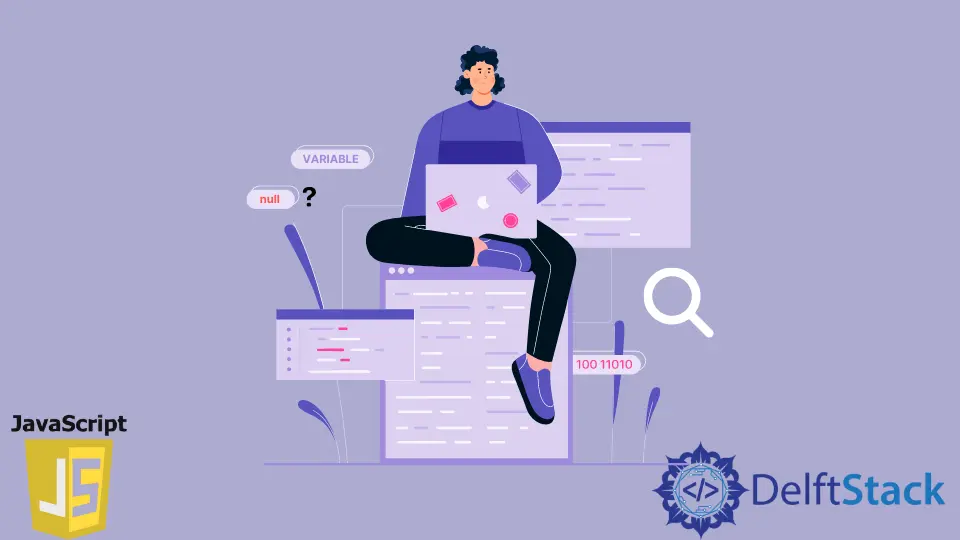
This tutorial teaches how to check if a variable is not null in JavaScript.
the Incorrect Way That Seems Right
if (myVar) {
...
}
This way will evaluate to true
when myVar
is null
, but it will also get executed when myVar
is any of these:
undefined
null
0
""
(the empty string)false
NaN
the Correct Way to Check Variable Is Not Null
if (myVar !== null) {
...
}
The above code is the right way to check if a variable is null
because it will be triggered only in one condition - the variable is actually null
.
Author: Harshit Jindal
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedInRelated Article - JavaScript Variable
- How to Access the Session Variable in JavaScript
- How to Check Undefined and Null Variable in JavaScript
- How to Mask Variable Value in JavaScript
- Why Global Variables Give Undefined Values in JavaScript
- How to Declare Multiple Variables in a Single Line in JavaScript
- How to Declare Multiple Variables in JavaScript