JavaScript href Expression
-
JavaScript
href
Expression -
Use
href
Expression With a Hash in JavaScript -
Use
href
Expression With a Function in JavaScript
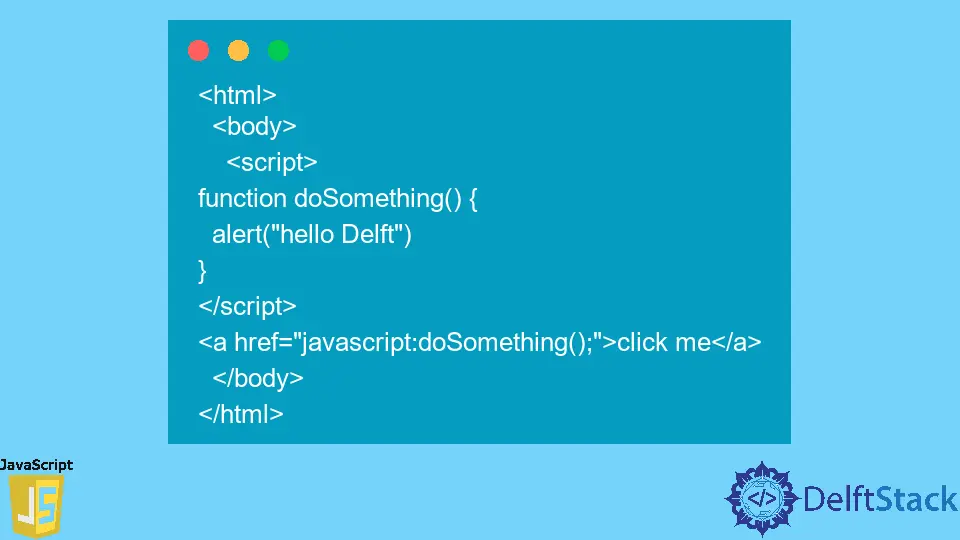
This article will help you understand a href
expression and its working mechanism in JavaScript.
JavaScript href
Expression
The href
property on the HTML element (or anchor element) generates a hyperlink to web pages, files, email addresses, page positions, or anything else a URL can represent.
<p>You can reach to this link:</p>
<ul>
<li><a href="https://jsfiddle.net/">JSFiddle</a></li>
</ul>
Output:
To remain on the same page, you can also provide a name or an empty URL in the href
attribute.
<a href="javascript:;"></a>
The code above is commonly used to create a link without specifying a true URL in the href
property.
Use href
Expression With a Hash in JavaScript
A hash #
specifies an HTML element id where the window should be dragged within a hyperlink.
Although href="#"
does not offer an id name, it does specify a location - the top of the page. The scroll position is moved to the top when you click an anchor with href="#"
.
<h1>Scroll to the bottom of the page and click the link</h1>
<a href="#">Scroll to Top</a>
You can see the demo here.
Use href
Expression With a Function in JavaScript
Basically, instead of using a link to move the page (or anchor), using this method will launch one or more JavaScript functions.
<html>
<body>
<script>
function doSomething() {
alert("hello Delft")
}
</script>
<a href="javascript:doSomething();">click me</a>
</body>
</html>
When you click the link, it will fire alert, call the JavaScript function, and you will see a popup window that says hello Delft
.
Output:
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn