The history.forward() Function in JavaScript
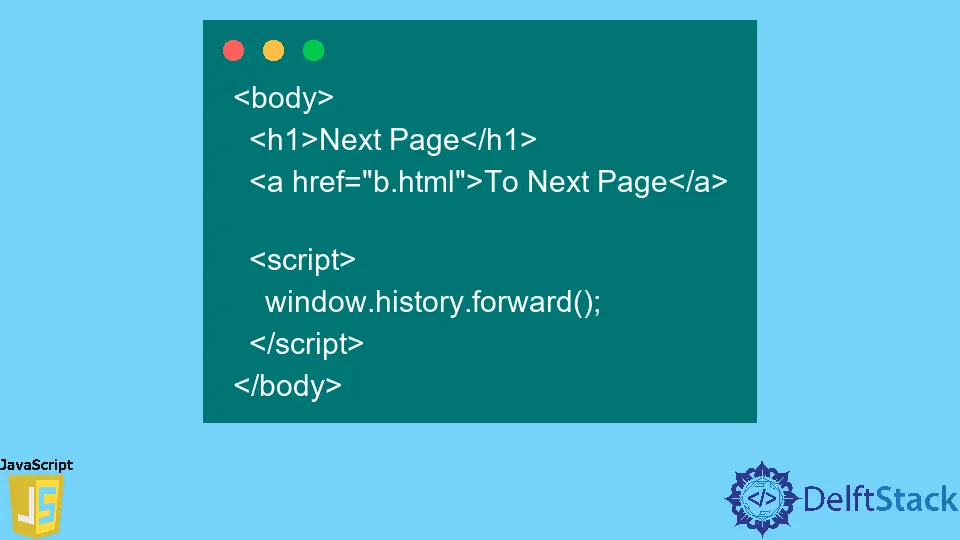
This article will show you how to navigate the browser using JavaScript.
Use the history.forward()
Function in JavaScript
The history object, which is always accessible as part of the browser’s window object, has a function called forward()
in JavaScript. The Forward
button on your browser is the same as using the history.forward()
function.
The method is called in an HTML page in the following way.
Example Code:
<body>
<h1>Next Page</h1>
<a href="b.html">To Next Page</a>
<script>
window.history.forward();
</script>
</body>
If your browser’s history does not include the next page, the history.forward()
function will have no effect.
The history.forward()
function is often used to deactivate the back button for online applications that need more robust security measures and move to the next page in the browser history. To do this, include the history.forward()
function on pages you don’t want users to return to.
Let’s take a scenario where you have two web pages, a.html
and b.html
, and you can access b.html
from a.html
.
Example Code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Document</title>
</head>
<body>
<h1>Next Page</h1>
<a href="b.html">To Next Page</a>
<script>
window.history.forward();
</script>
</body>
</html>
The following script may be added to the a
page if you don’t want the user to return from the b
page to the a
page.
Script:
<script>window.history.forward();</script>
First, make two web pages like a.html
and b.html
and save both files. Ensure the code is on the main page to redirect to another page like b.html
.
Output:
When you click on the link(To Next Page
), it will redirect you to the next html/page.
When you return to the a
page from the b
page, the history.forward()
function is automatically invoked since there is no method to deactivate the back button on the browser using JavaScript.
This approach has been used by specific programs that need to prevent users from leaving the current page and returning.
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedIn