How to Group an Array of Objects in JavaScript
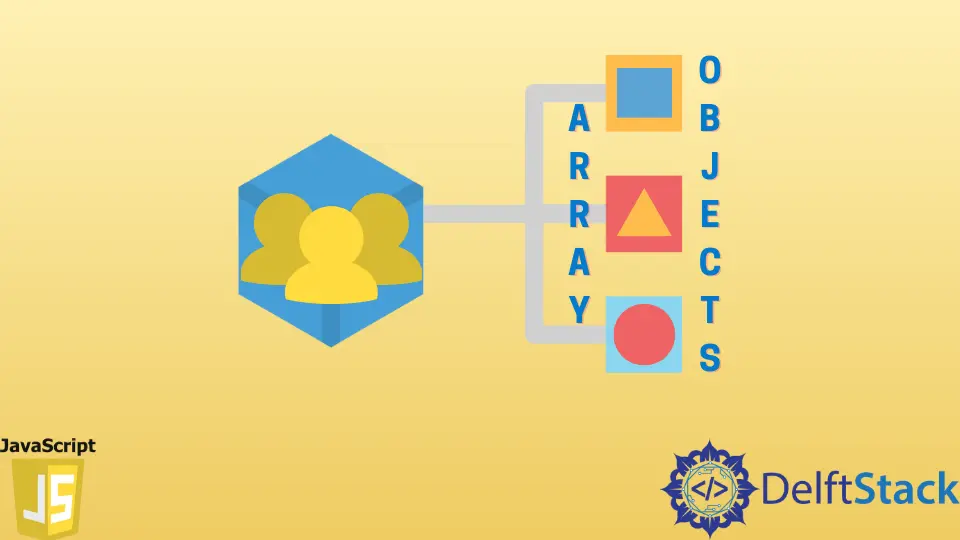
This article will demonstrate the most efficient method to group an array of objects in JavaScript.
Use reduce()
to Group an Array of Objects in JavaScript
The most efficient way to group an array of objects in JavaScript is to use the reduce()
function. This method executes each array element’s (specified) reducer function and produces a single output value.
Example:
We have a product list with the two properties, name and category.
const products = [
{name: 'Mathematics', category: 'Subject'},
{name: 'Cow', category: 'Animal'},
{name: 'Science', category: 'Subject'},
];
In the above example, products
is an array of objects.
Next, we need to perform a simple operation. Using the list of products, we will group products by category.
const groupByCategory = {
Subjects: [
{name: 'Mathematics', category: 'Subjects'},
{name: 'Science', category: 'Subjects'},
],
Animals: [{name: 'Cow', category: 'Animals'}],
};
The usual way to get an array-like groupByCategory
from an array of products is to call the array.reduce()
method with the appropriate callback function.
const groupByCategory = products.reduce((group, product) => {
const {category} = product;
group[category] = group[category] ?? [];
group[category].push(product);
return group;
}, {});
Output:
"{
'Subject': [
{
'name': 'Mathematics',
'category': 'Subject'
},
{
'name': 'Science',
'category': 'Subject'
}
],
'Animal': [
{
'name': 'Cow',
'category': 'Animal'
}
]
}"
The products.reduce()
function reduces the array to product objects grouped by category.
The array.groupBy
(callback) accepts a callback function invoked with three arguments: the current array element, the index, and the array itself. The callback must return a string (the group name to which you want to add the feature).
const groupedObject = array.groupBy((item, index, array) => {
...return groupNameAsString;
});
Shiv is a self-driven and passionate Machine learning Learner who is innovative in application design, development, testing, and deployment and provides program requirements into sustainable advanced technical solutions through JavaScript, Python, and other programs for continuous improvement of AI technologies.
LinkedInRelated Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript