How to Declare Global Variables in JavaScript
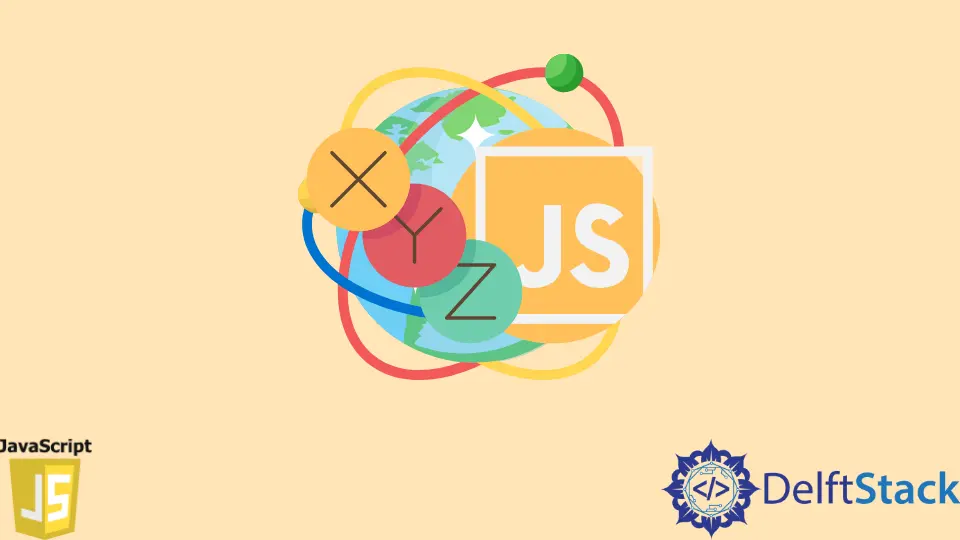
This tutorial introduces how to declare global variables in JavaScript.
Variables hold the data and information, which can be edited anytime. In JavaScript, the variables can be declared using keywords such as const
, let
, and var
. The scope of the variable is usually defined by the place of their declaration. A variable declared inside a function has local scope and hence is called a local variable. Global variables are defined outside the functions and have access to all functions and not just one.
We can also declare global variables inside a function by using the window
keyword. The lifetime of a Global Variable ends when we close the browser window, unlike local variables that get deleted when the function ends. Global variables often are troublesome to cause debugging problems and hence are generally avoided. They can be modified by any function, which makes life miserable.
Declare Global Variable in JavaScript
<script>
var yourGlobalVariable;
function foo() {
// ...
}
</script>
We can declare a global variable by declaring a variable outside all functions.
Declare Global Variables Inside a JavaScript Function
Use globalThis
to Declare Global Variables in JavaScript
<script>
function foo() {
globalThis.yourGlobalVariable = ...;
}
</script>
The global globalThis
property contains the global this
value, which is akin to the global object and can be used to declare global variables inside a function.
Use window
to Declare Global Variables in JavaScript
<script>
function foo() {
window.yourGlobalVariable = ...;
}
</script>
In browsers, all the global variables declared with var
are properties of the window
object. We can declare global variables inside a function by attaching properties to a window
object.
Harshit Jindal has done his Bachelors in Computer Science Engineering(2021) from DTU. He has always been a problem solver and now turned that into his profession. Currently working at M365 Cloud Security team(Torus) on Cloud Security Services and Datacenter Buildout Automation.
LinkedInRelated Article - JavaScript Variable
- How to Access the Session Variable in JavaScript
- How to Check Undefined and Null Variable in JavaScript
- How to Mask Variable Value in JavaScript
- Why Global Variables Give Undefined Values in JavaScript
- How to Declare Multiple Variables in a Single Line in JavaScript
- How to Declare Multiple Variables in JavaScript