How to Get the Last Item in an Array in JavaScript
Kirill Ibrahim
Feb 02, 2024
-
Use the
length
Property to Get the Last Item in an Array in JavaScript -
Use the
pop()
Method to Get the Last Item in an Array in JavaScript
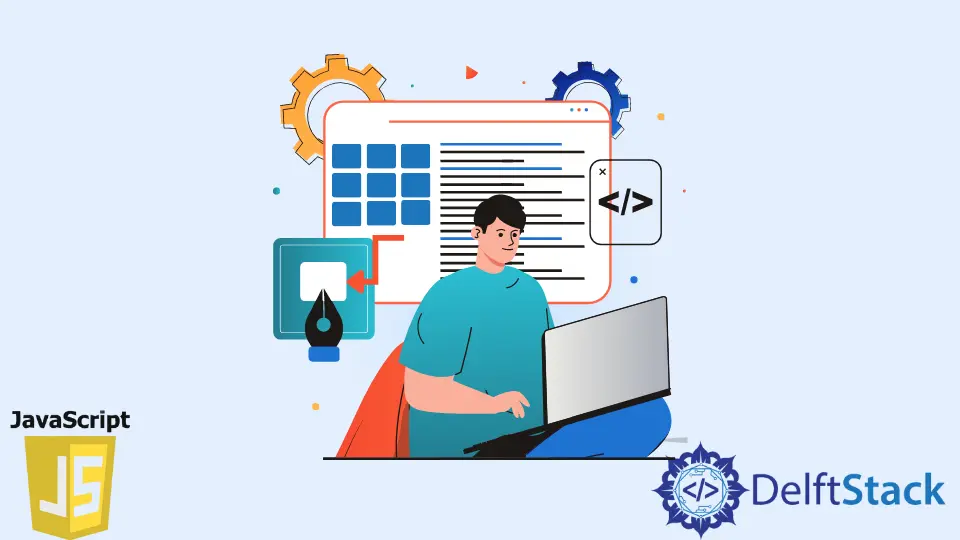
The array is used to store multiple values at a time. JavaScript has different methods to get the last item in an array. Every method below will have a code example, which you can run on your machine.
Use the length
Property to Get the Last Item in an Array in JavaScript
We can access the last item in the array by using the length property.
Example of the length
Property
let arr1 = [0, 2, 1, 5, 8];
const getLastArrItem = (arr) => {
let lastItem = arr[arr.length - 1];
console.log(`Last element is ${lastItem}`);
} getLastArrItem(arr1);
Use the pop()
Method to Get the Last Item in an Array in JavaScript
The array.pop()
function is a built-in function in JavaScript. It is used to return and remove the last element of the array. If we do not want to mutate our array, I do not recommend this way.
Example of the pop()
Method
let arr1 = [0, 2, 1, 5, 8];
const getLastArrItem = (arr) => {
let lastItem = arr.pop();
console.log(`Last element is ${lastItem}`);
console.log(`Our array after remove last item: ${arr}`);
} getLastArrItem(arr1);
Related Article - JavaScript Array
- How to Check if Array Contains Value in JavaScript
- How to Create Array of Specific Length in JavaScript
- How to Convert Array to String in JavaScript
- How to Remove First Element From an Array in JavaScript
- How to Search Objects From an Array in JavaScript
- How to Convert Arguments to an Array in JavaScript